Animation Instance
새로운 cpp인 AnimInstance를 상속받는 ShooterAnimInstance를 생성해줍니다.
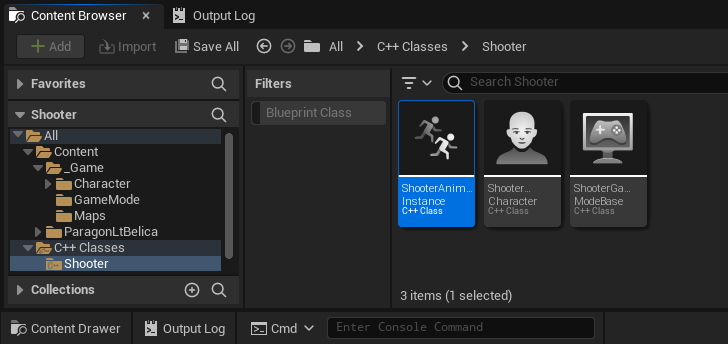
ShooterAnimInstance.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Animation/AnimInstance.h"
#include "ShooterAnimInstance.generated.h"
UCLASS()
class SHOOTER_API UShooterAnimInstance : public UAnimInstance
{
GENERATED_BODY()
public:
UFUNCTION(BlueprintCallable)
void UpdateAnimationProperties(float Deltatime);
virtual void NativeInitializeAnimation() override;
private:
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
class AShooterCharacter *ShooterCharacter;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
float Speed;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
bool bIsInAir;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
bool bIsAcceleerating;
};
- UpdateAnimationProperties : 애니메이션 속성 업데이트를 해주는 사용자 정의 함수입니다.
- NativeInitializeAnimation : 언리얼 엔진에서 애니메이션 인스턴스를 초기화하는 데 사용되는 함수입니다.
- ShooterCharacter : 이 애니메이션을 소유하는 AShooterCharacter입니다.
- Speed : ShooterCharacter의 속도를 가집니다.
- bIsInAir : ShooterCharacter의 공중여부를 가집니다.
- bIsAcceleerating : ShooterCharacter의 가속여부(속도가 0이상 인지)를 가집니다.
ShooterAnimInstance.cpp
// ShooterAnimInstance.cpp
#include "ShooterAnimInstance.h"
#include "ShooterCharacter.h"
#include "GameFramework/CharacterMovementComponent.h"
void UShooterAnimInstance::NativeInitializeAnimation()
{
ShooterCharacter = Cast<AShooterCharacter>(TryGetPawnOwner());
}
void UShooterAnimInstance::UpdateAnimationProperties(float Deltatime)
{
if (ShooterCharacter == nullptr)
{
ShooterCharacter = Cast<AShooterCharacter>(TryGetPawnOwner());
}
if (ShooterCharacter)
{
// Get the speed of the character from velocity
FVector Velocity{ShooterCharacter->GetVelocity()};
Velocity.Z = 0;
Speed = Velocity.Size();
// Is the character in the air?
bIsInAir = ShooterCharacter->GetCharacterMovement()->IsFalling();
// Is the character accelerating?
if (ShooterCharacter->GetCharacterMovement()->GetCurrentAcceleration().Size() > 0.f)
{
bIsAcceleerating = true;
}
else
{
bIsAcceleerating = false;
}
}
}
- ShooterCharacter = Cast<AShooterCharacter>(TryGetPawnOwner())
- TryGetPawnOwner() : 애니메이션 인스턴스가 현재 연결된 Pawn 오브젝트에 대한 참조를 시도하여 반환합니다.
- ShooterCharacter->GetVelocity() : Pawn의 속도를 FVector로 반환합니다.
- Velocity.Z = 0 : 점프 및 낙하를 속도에서 제외시킵니다.
- Speed = Velocity.Size() : 속도의 절대값을 구합니다.
- bIsInAir = ShooterCharacter->GetCharacterMovement()->IsFalling() : 공중에 존재하는지 Bool 값을 반환합니다.
- ShooterCharacter->GetCharacterMovement()->GetCurrentAcceleration().Size() > 0.f :
- GetCharacterMovement : ACharacter 클래스의 인스턴스에 연결된 UCharacterMovementComponent를 반환하는 함수입니다.
- GetCurrentAcceleration : UCharacterMovementComponent에서 현재 캐릭터의 가속도를 반환하는 함수입니다. 이 값은 캐릭터가 얼마나 빠르게 속도를 증가시키고 있는지를 나타냅니다.
ABP_ShooterCharacter를 만들기고 BP_ShooterCharacter에서 할당하기
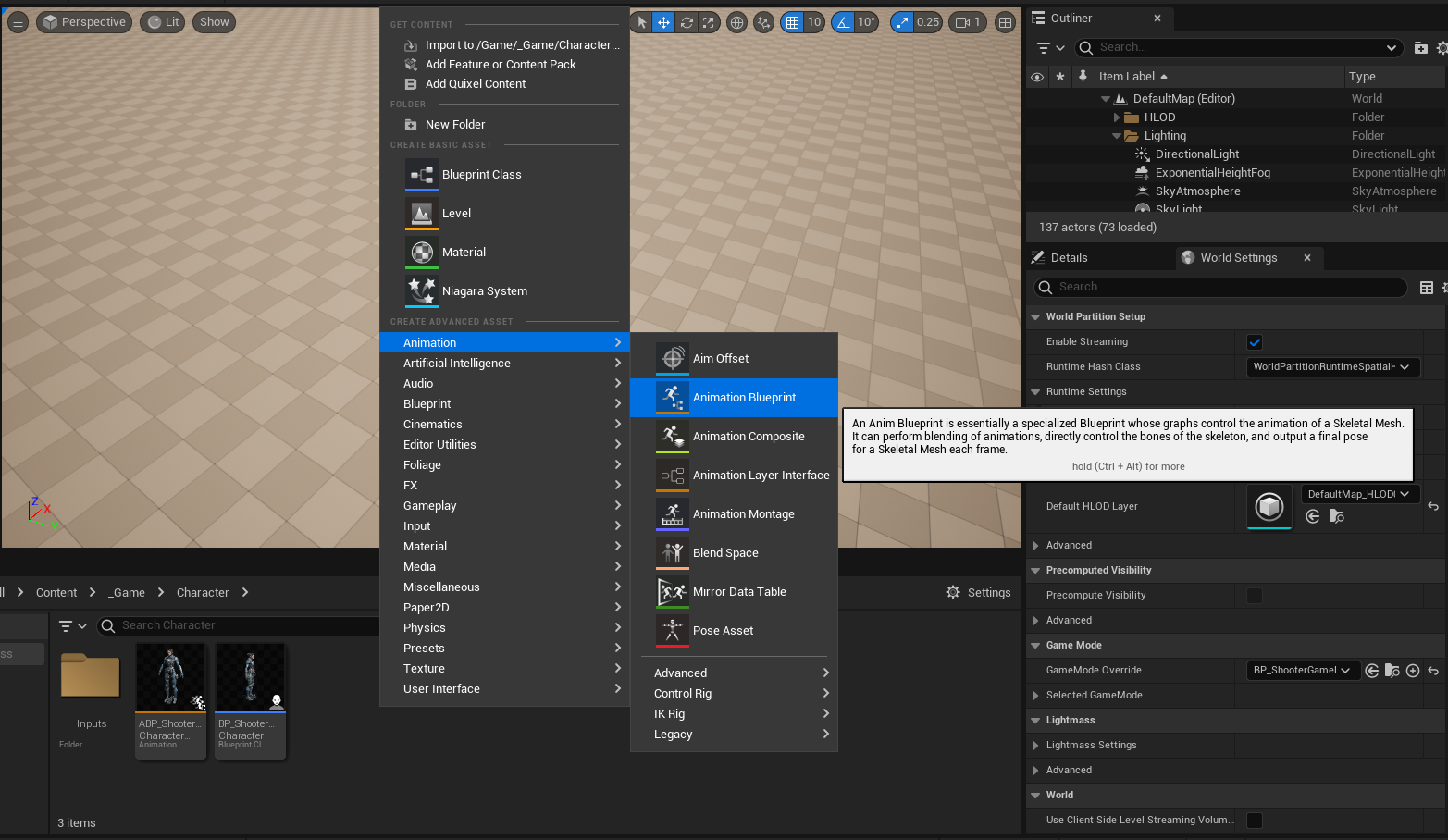
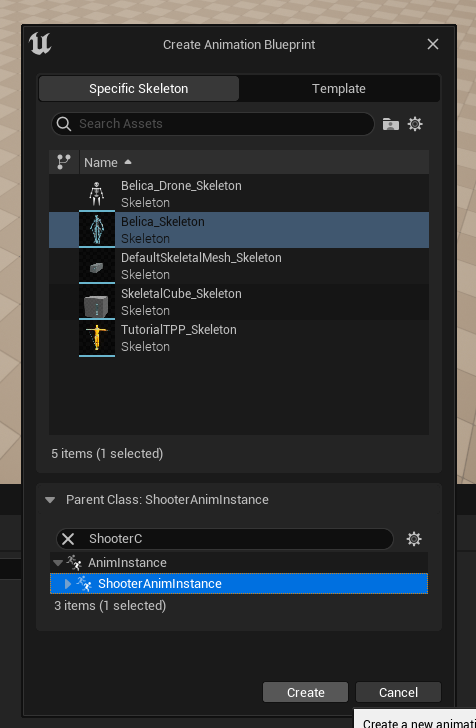
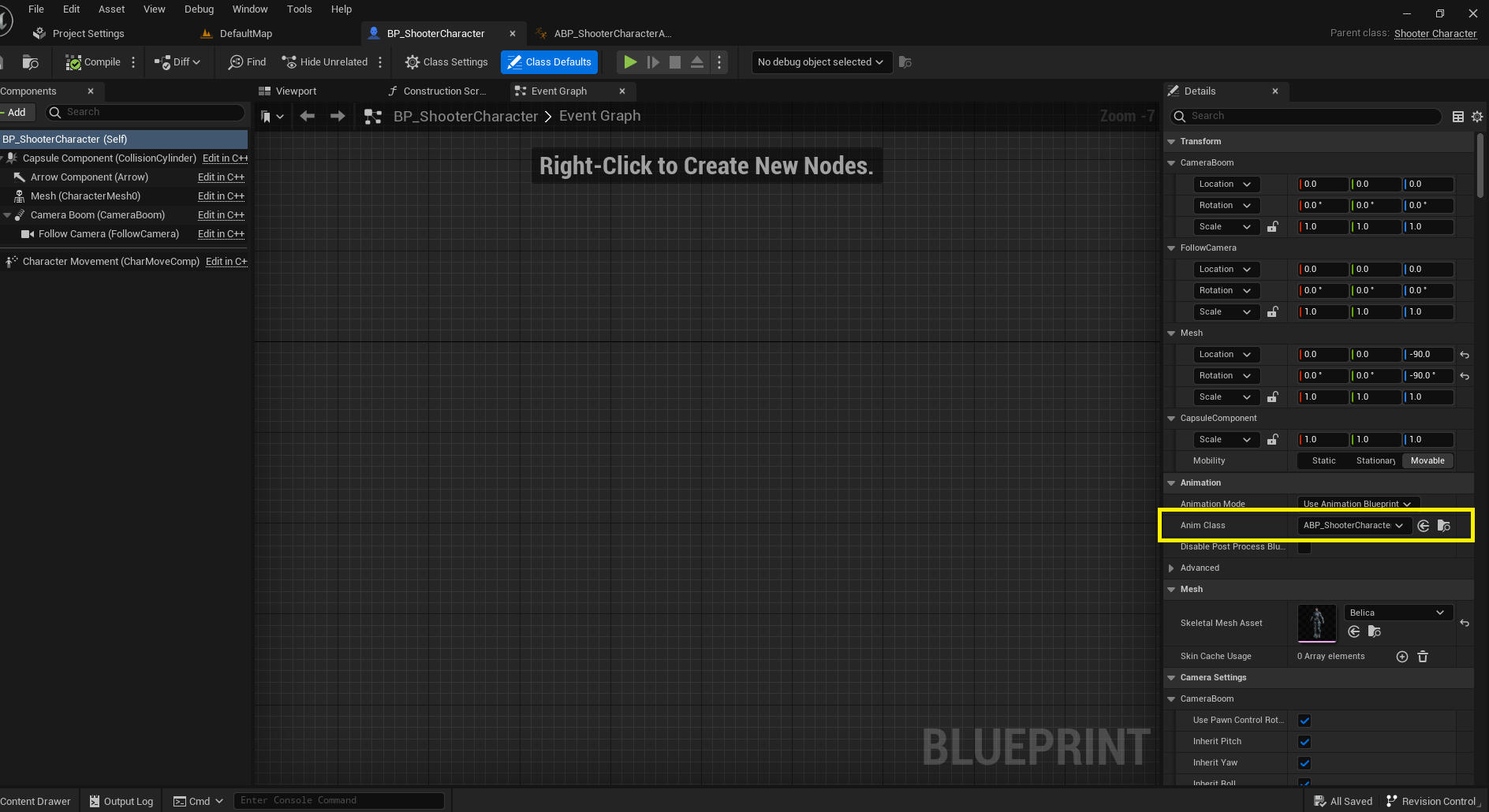
Event Graph에서 Update Animation Properties를 Event Blueprint Update Animation(애니메이션의 각 프레임을 업데이트할 때마다 자동으로 호출)과 연결

Run Animation
State Machine으로 노드를 생성합니다. (이름은 Ground Locomotion으로 수정)
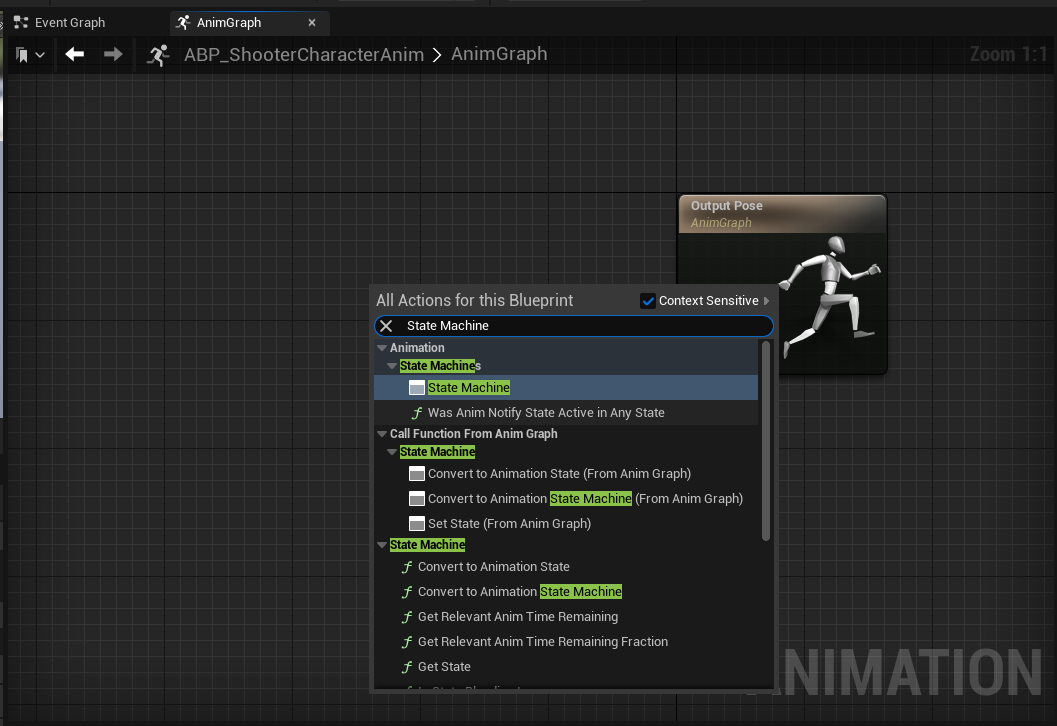
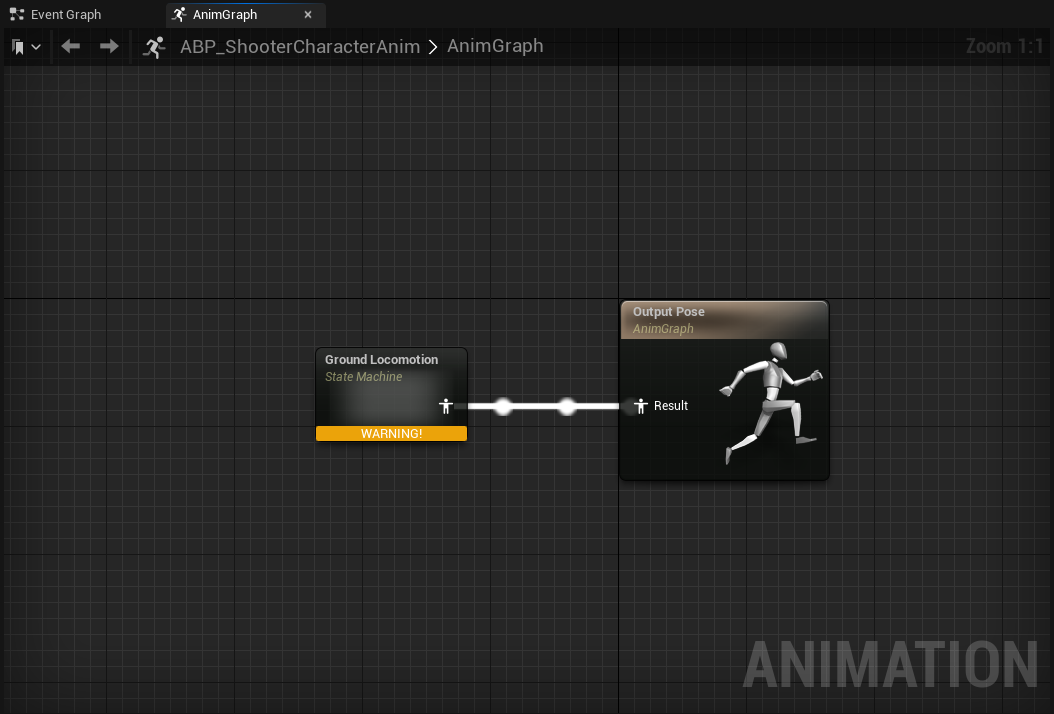
Ground Locomotion에 들어가서 Add State로 사진과 같이 그래프를 만들어 줍니다.

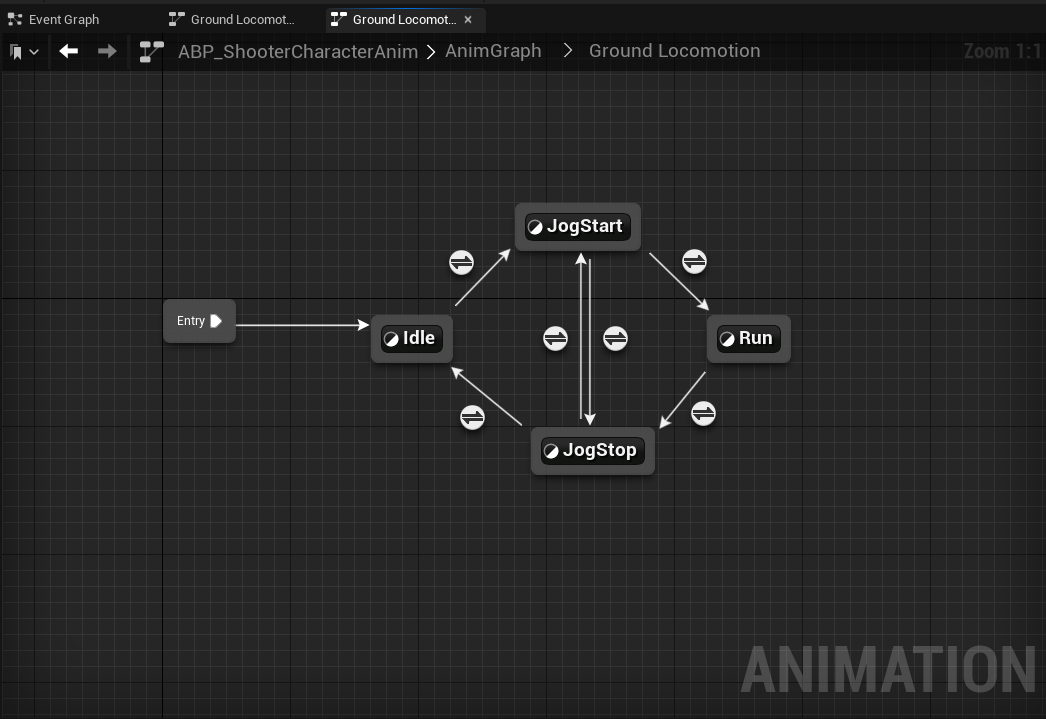
Idle State에 들어가서 좌측 하단의 Asset Browser에서 Idle_Relaced를 찾아 드래그해서 설정. 나머지는 다음 사진과 맞게 설정해 줍니다.
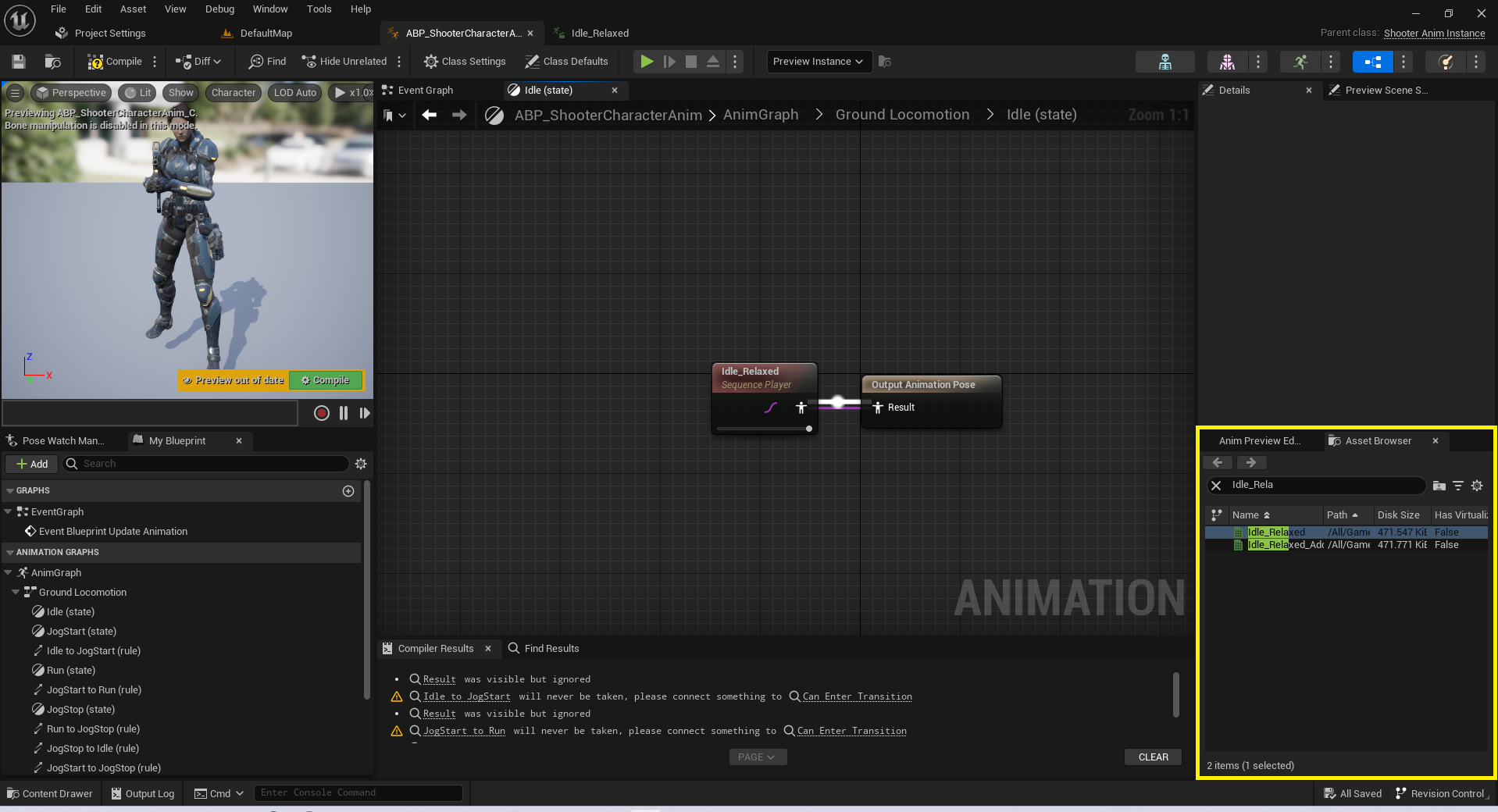
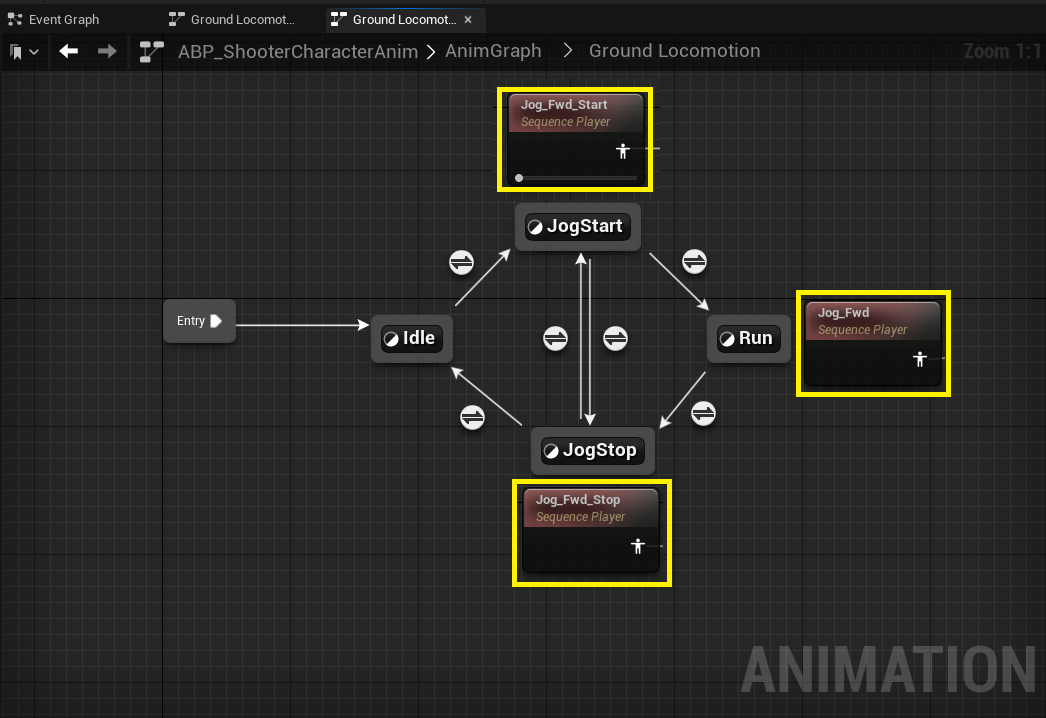
Idle과 JogStart를 연결해주는 선분위의 버튼을 눌러서 변환조건을 설정해준다.
(Speed가 0보다 크고 공중에 있지 않으며, 가속도가 있을때 달리기 시작한다.)
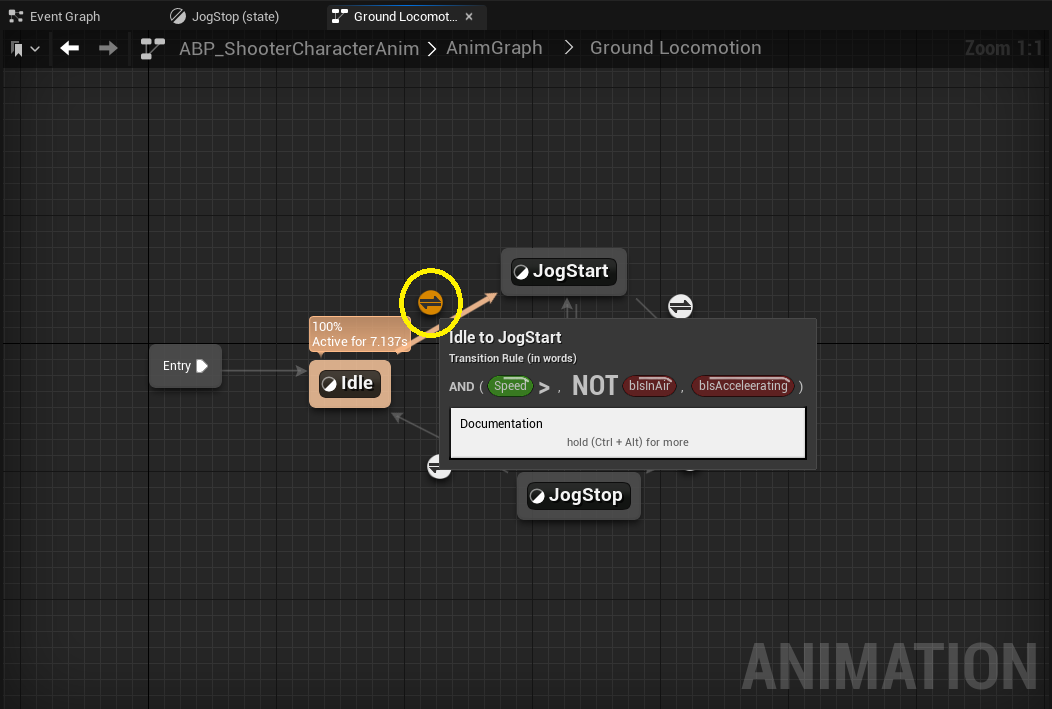
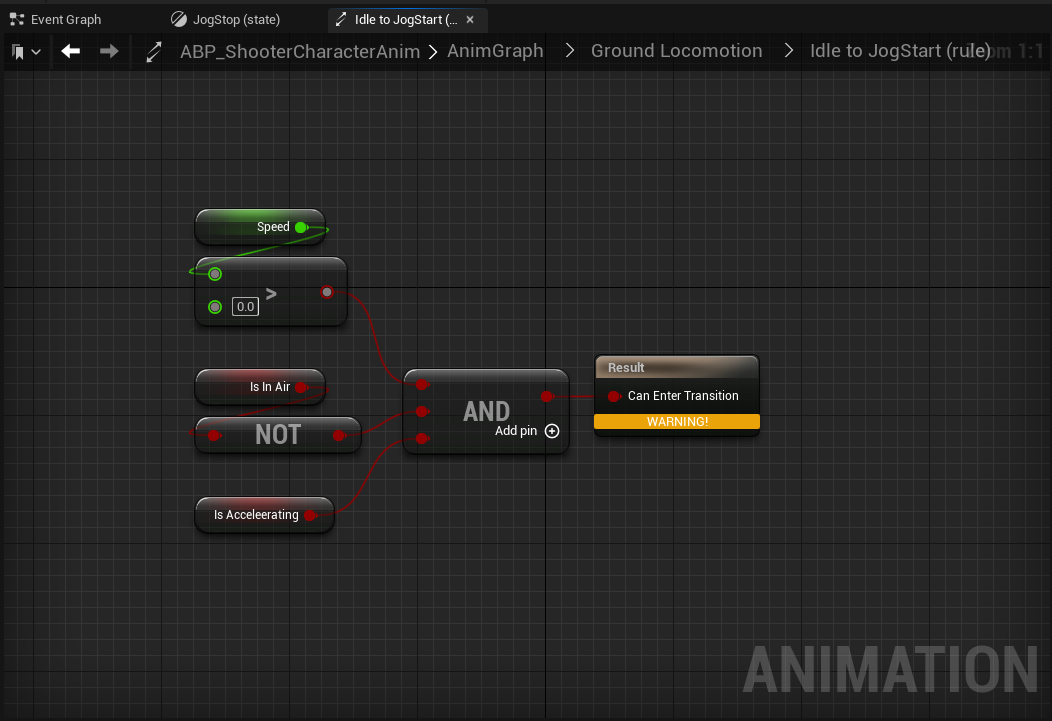
JogStart에서 Run으로 넘어가는 조건을 한번 클릭하면 Details를 수정할 수 있는데, Automatic Rule Based on Sequence Player in State를 True로 하면 애니메이션이 끝난 후 자동으로 전환된다.
(Duration은 0.8로 하는게 부드럽다는 영상의 조언에 따르겠다.)
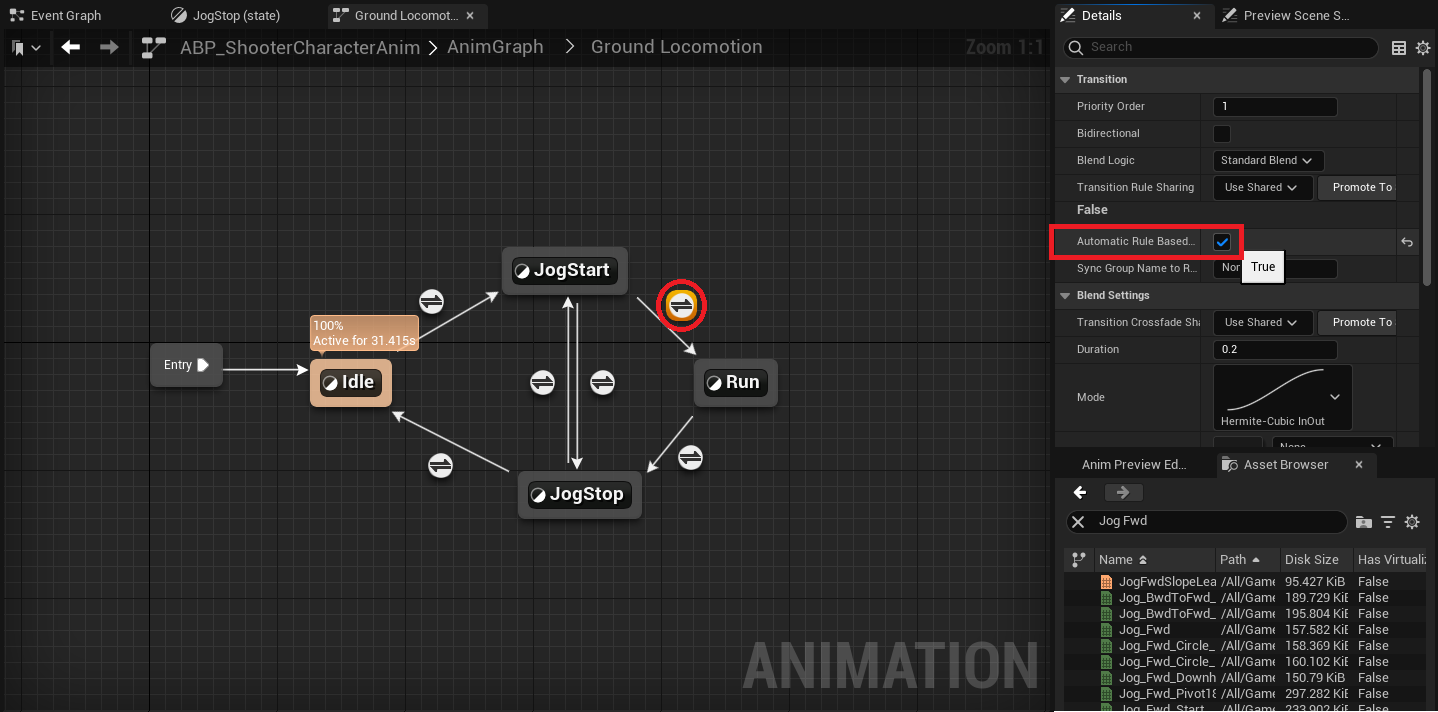
아래와 같이 설정해 줍니다.
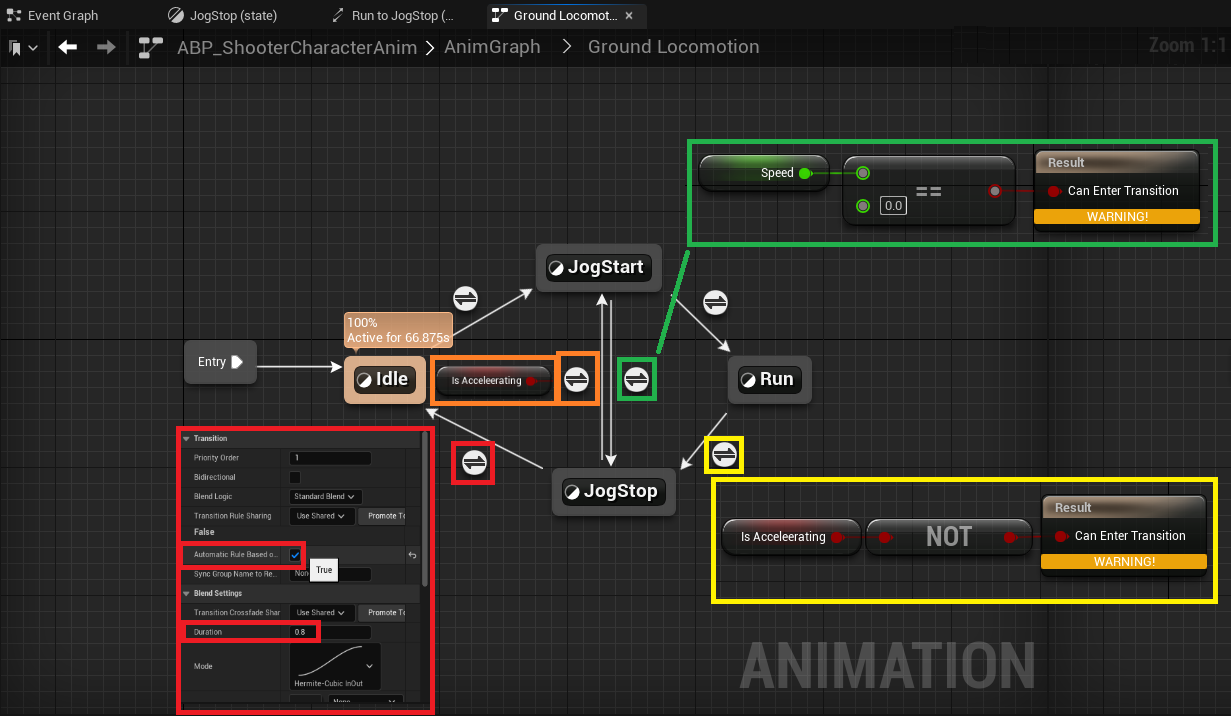
Run에 있는 Jog_Fwd에서 Detail의 Loop Animation을 True로 바꿔줘야 달리기를 계속할 수 있습니다.
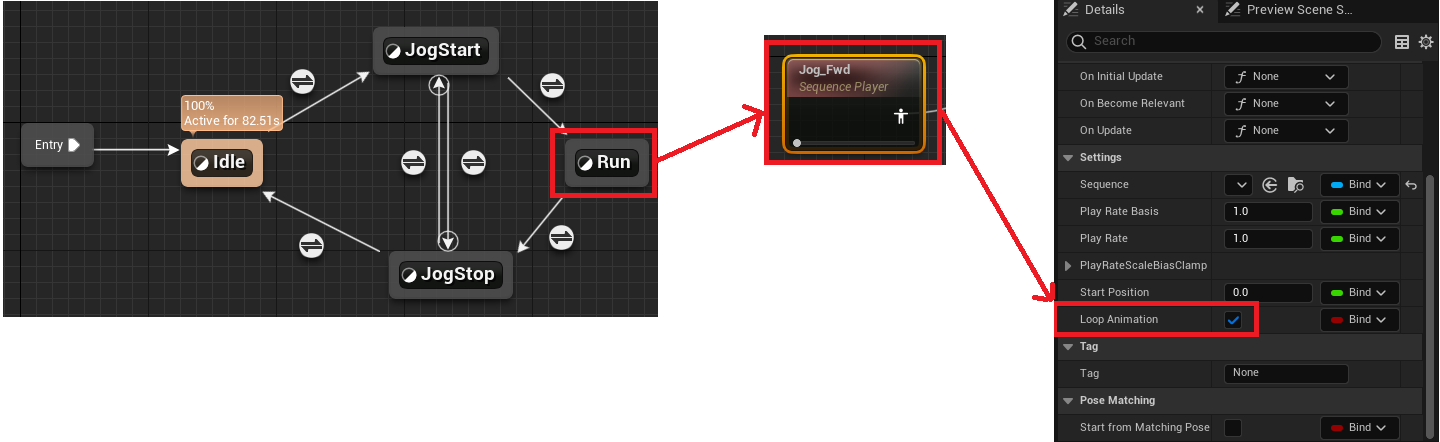
Trimming Animation
애니메이션의 수정을 할것인데 원본을 보호하기 위해서 새로운 폴더를 만들고 복사를 해줍니다.
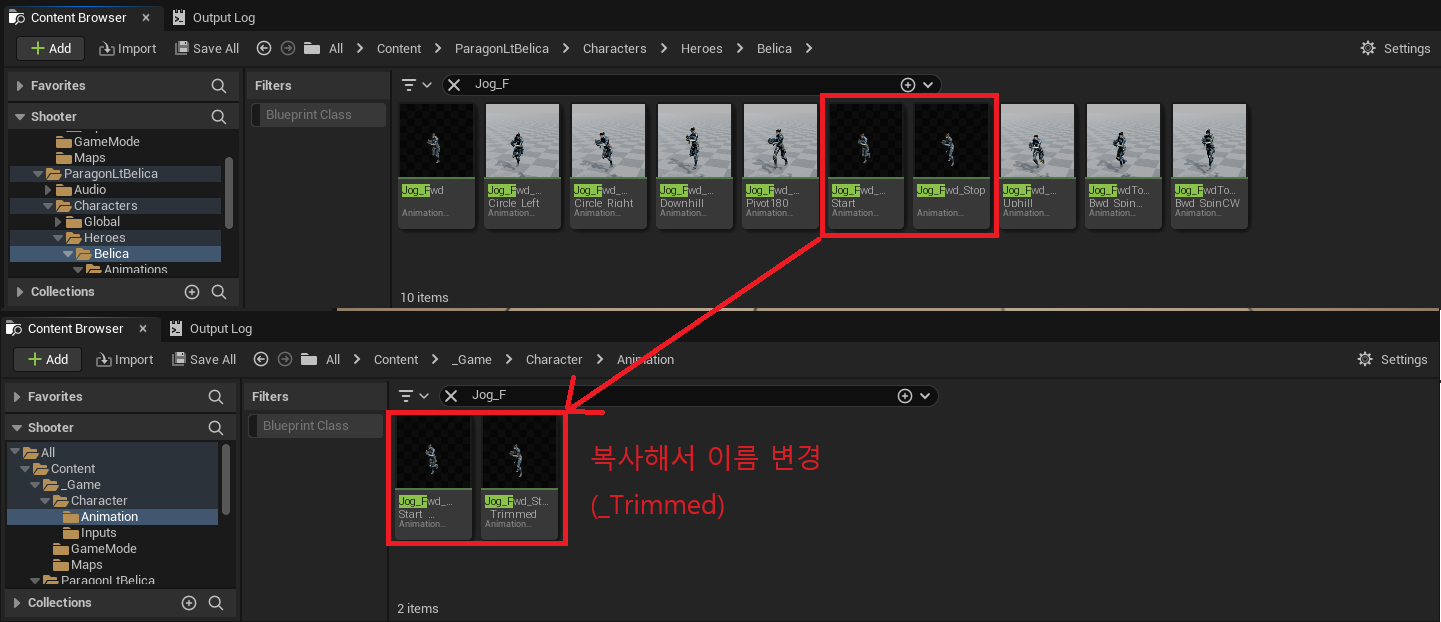
Jog_Fwd_Start_Trimmed를 수정해 줍니다.

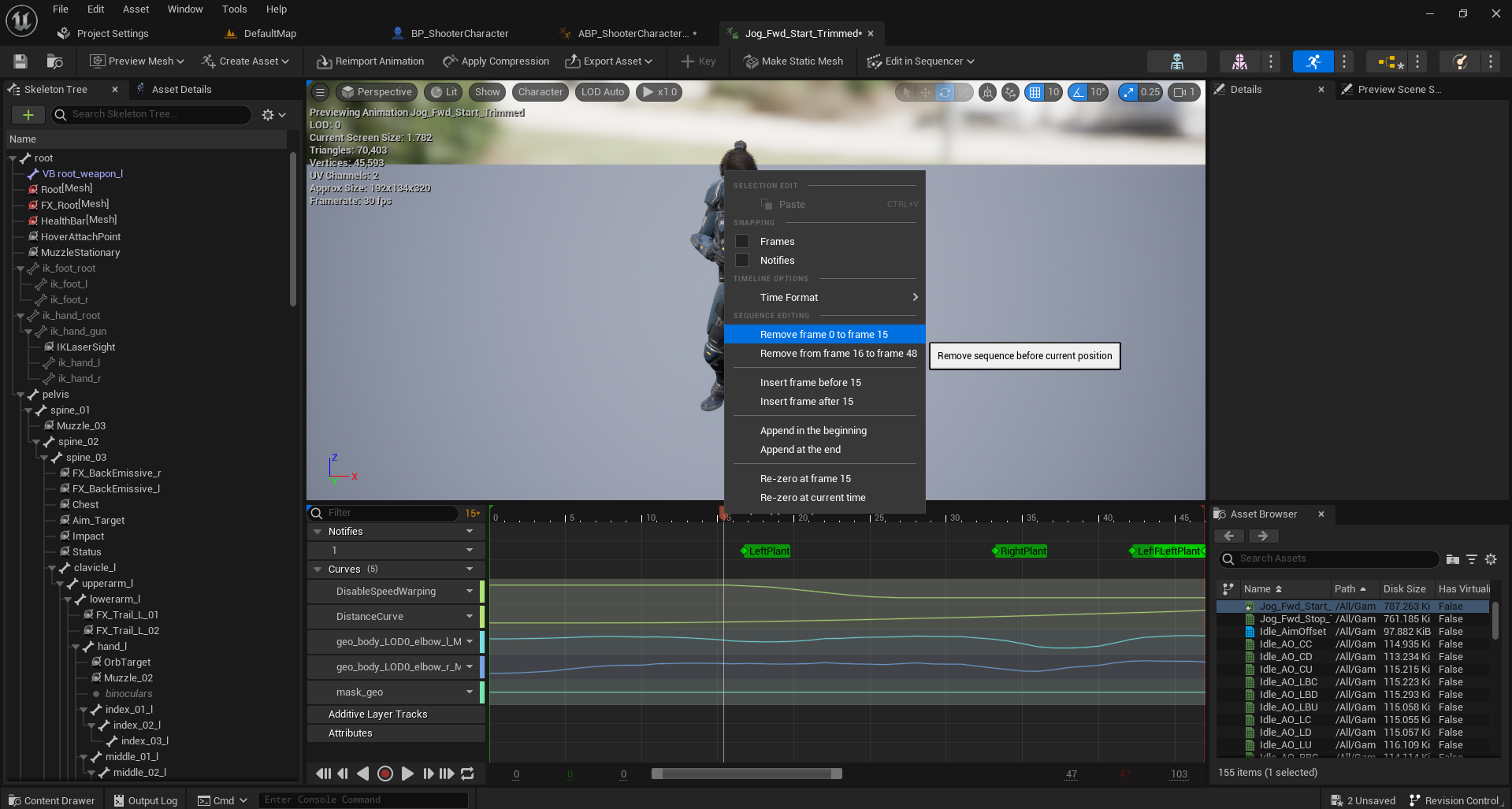
Jog_Fwd_End_Trimmed를 수정해 줍니다.
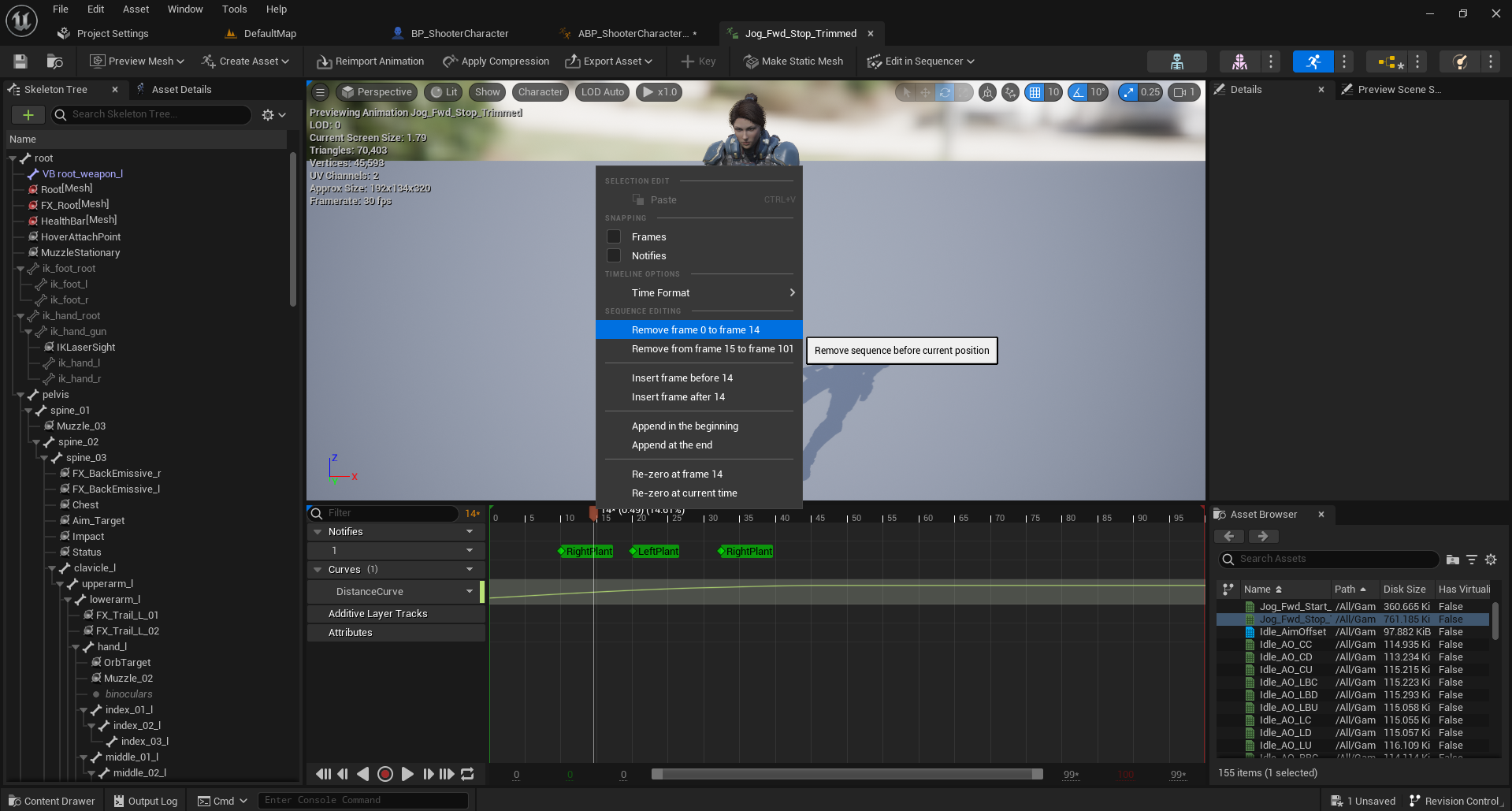
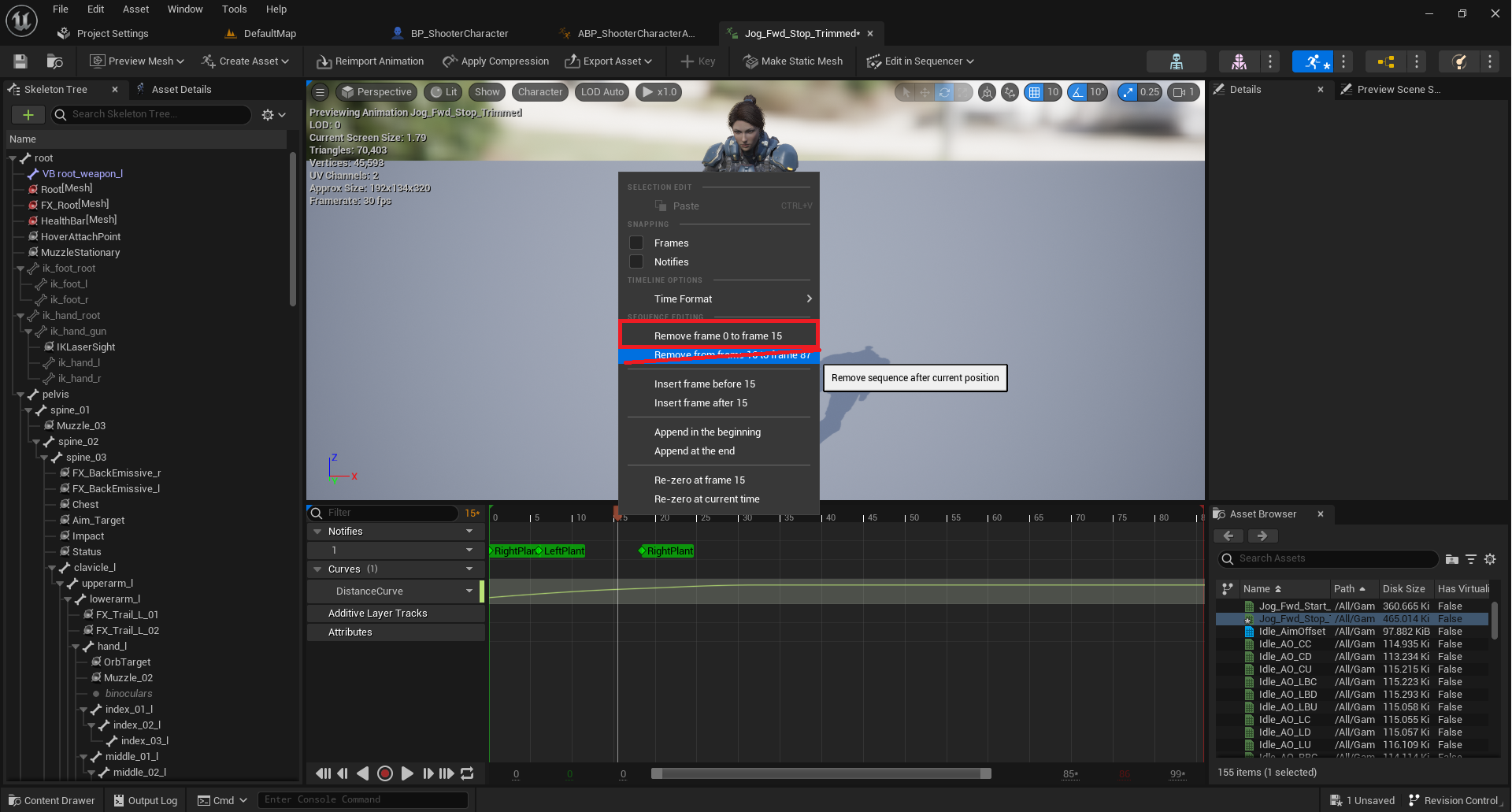
Rotate Character to Movement
ShooterCharacter.cpp 생성자에 이동 방향으로 Pawn 회전 방향을 정하고, 이동 관련 코드를 추가해줍니다.
AShooterCharacter::AShooterCharacter() : BaseTrunRate(45.f), BaseLookUpRate(45.f)
{
...
// 컨트롤러가 회전할 때 캐릭터가 회전하지 않도록 설정합니다. 컨트롤러는 오직 카메라에만 영향을 줍니다.
bUseControllerRotationPitch = false;
bUseControllerRotationYaw = false;
bUseControllerRotationRoll = false;
// 캐릭터 이동을 구성합니다
GetCharacterMovement()->bOrientRotationToMovement = true; // 캐릭터는 입력 방향으로 이동합니다
GetCharacterMovement()->RotationRate = FRotator(0.f, 540.f, 0.f); // 이 회전 속도로...
GetCharacterMovement()->JumpZVelocity = 600.f;
GetCharacterMovement()->AirControl = 0.2f;
}
- bUseControllerRotationPitch, Yaw, Roll : Pawn의 회전을 컨트롤러 회전으로 할 지 선택합니다.
- bOrientRotationToMovement : 회전 방향을 이동 방향으로 할 지 선택합니다.
- RotationRate = FRotator(0.f, 540.f, 0.f) : 캐릭터가 얼마나 빠르게 회전할 수 있는지를 정의합니다.
- 초당 540도의 속도로 Yaw 축(좌우 방향)을 중심으로 회전할 수 있음을 의미합니다.
- JumpZVelocity = 600.f : 캐릭터가 점프할 때의 수직 속도(즉, Z축 방향 속도)를 설정합니다.
- AirControl = 0.2f : 캐릭터가 공중에 있을 때 얼마나 많은 이동 제어를 할 수 있는지를 나타냅니다.
- 값이 0에 가까울수록 공중에서의 제어가 줄어들고, 1에 가까울수록 더 많은 제어를 할 수 있습니다.
- bOrientRotationToMovement와 RotationRate 설정은 캐릭터의 회전을 부드럽고 직관적으로 만들어주는 핵심 요소입니다.
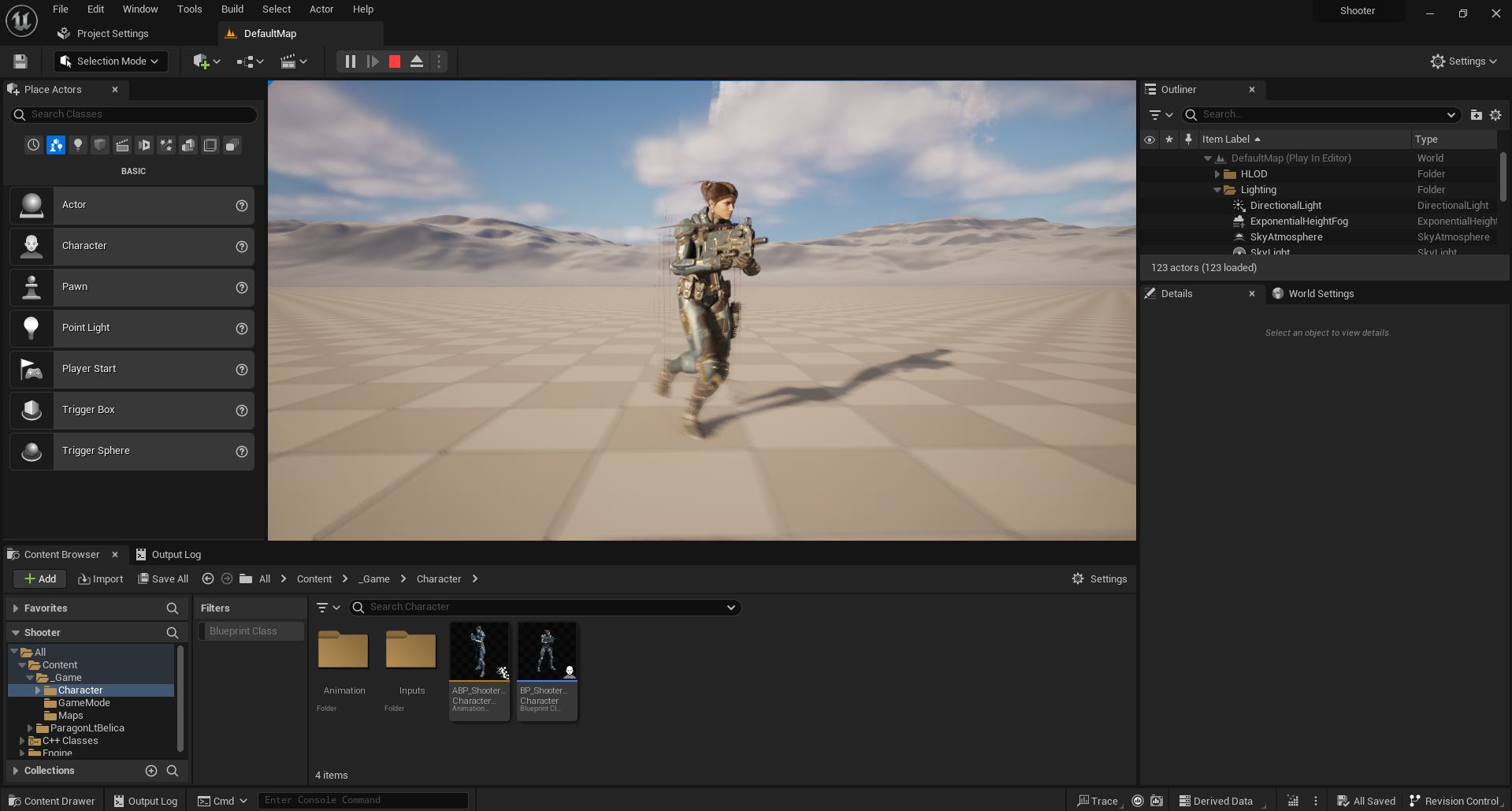
UE5를 사용하면서 회전(bOrientRotationToMovement = true)이 되지 않는 문제.
영상에서는 CPP 파일 수정후 회전이 작동하지 않을경우 BP를 확인하라 합니다. 허나 BP의 값을 수정해도 움직이지 않을경우 BP의 RotationRate 설정에 Yaw가 540으로 되어있는지 확인해 줍시다. (BP에서는 XYZ순으로 보여주기 때문에 3번째 매개변수가 540이어야 합니다.
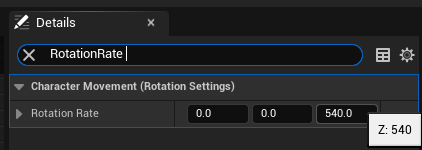
'Unreal 공부 > Unreal Engine 4 C++ The Ultimate Shooter' 카테고리의 다른 글
Animation - 5 (1) | 2023.12.31 |
---|---|
Animation - 4 (1) | 2023.12.31 |
Animation - 3 (1) | 2023.12.30 |
Animation - 2 (1) | 2023.12.28 |
[UE] C++에서 SpringArm과 Camera 구현하기 (1) | 2023.12.22 |
Animation Instance
새로운 cpp인 AnimInstance를 상속받는 ShooterAnimInstance를 생성해줍니다.
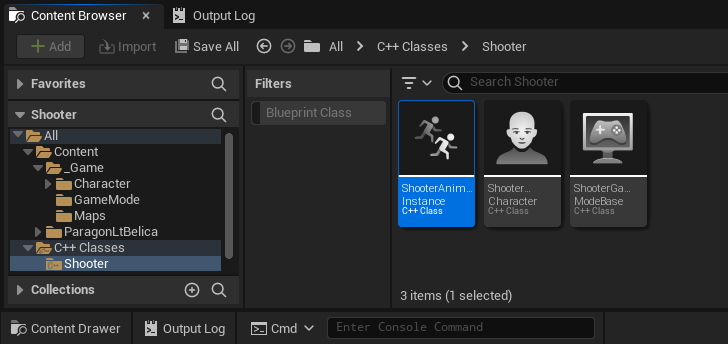
ShooterAnimInstance.h
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "Animation/AnimInstance.h"
#include "ShooterAnimInstance.generated.h"
UCLASS()
class SHOOTER_API UShooterAnimInstance : public UAnimInstance
{
GENERATED_BODY()
public:
UFUNCTION(BlueprintCallable)
void UpdateAnimationProperties(float Deltatime);
virtual void NativeInitializeAnimation() override;
private:
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
class AShooterCharacter *ShooterCharacter;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
float Speed;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
bool bIsInAir;
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Movement, meta = (AllowPrivateAccess = "true"))
bool bIsAcceleerating;
};
- UpdateAnimationProperties : 애니메이션 속성 업데이트를 해주는 사용자 정의 함수입니다.
- NativeInitializeAnimation : 언리얼 엔진에서 애니메이션 인스턴스를 초기화하는 데 사용되는 함수입니다.
- ShooterCharacter : 이 애니메이션을 소유하는 AShooterCharacter입니다.
- Speed : ShooterCharacter의 속도를 가집니다.
- bIsInAir : ShooterCharacter의 공중여부를 가집니다.
- bIsAcceleerating : ShooterCharacter의 가속여부(속도가 0이상 인지)를 가집니다.
ShooterAnimInstance.cpp
// ShooterAnimInstance.cpp
#include "ShooterAnimInstance.h"
#include "ShooterCharacter.h"
#include "GameFramework/CharacterMovementComponent.h"
void UShooterAnimInstance::NativeInitializeAnimation()
{
ShooterCharacter = Cast<AShooterCharacter>(TryGetPawnOwner());
}
void UShooterAnimInstance::UpdateAnimationProperties(float Deltatime)
{
if (ShooterCharacter == nullptr)
{
ShooterCharacter = Cast<AShooterCharacter>(TryGetPawnOwner());
}
if (ShooterCharacter)
{
// Get the speed of the character from velocity
FVector Velocity{ShooterCharacter->GetVelocity()};
Velocity.Z = 0;
Speed = Velocity.Size();
// Is the character in the air?
bIsInAir = ShooterCharacter->GetCharacterMovement()->IsFalling();
// Is the character accelerating?
if (ShooterCharacter->GetCharacterMovement()->GetCurrentAcceleration().Size() > 0.f)
{
bIsAcceleerating = true;
}
else
{
bIsAcceleerating = false;
}
}
}
- ShooterCharacter = Cast<AShooterCharacter>(TryGetPawnOwner())
- TryGetPawnOwner() : 애니메이션 인스턴스가 현재 연결된 Pawn 오브젝트에 대한 참조를 시도하여 반환합니다.
- ShooterCharacter->GetVelocity() : Pawn의 속도를 FVector로 반환합니다.
- Velocity.Z = 0 : 점프 및 낙하를 속도에서 제외시킵니다.
- Speed = Velocity.Size() : 속도의 절대값을 구합니다.
- bIsInAir = ShooterCharacter->GetCharacterMovement()->IsFalling() : 공중에 존재하는지 Bool 값을 반환합니다.
- ShooterCharacter->GetCharacterMovement()->GetCurrentAcceleration().Size() > 0.f :
- GetCharacterMovement : ACharacter 클래스의 인스턴스에 연결된 UCharacterMovementComponent를 반환하는 함수입니다.
- GetCurrentAcceleration : UCharacterMovementComponent에서 현재 캐릭터의 가속도를 반환하는 함수입니다. 이 값은 캐릭터가 얼마나 빠르게 속도를 증가시키고 있는지를 나타냅니다.
ABP_ShooterCharacter를 만들기고 BP_ShooterCharacter에서 할당하기
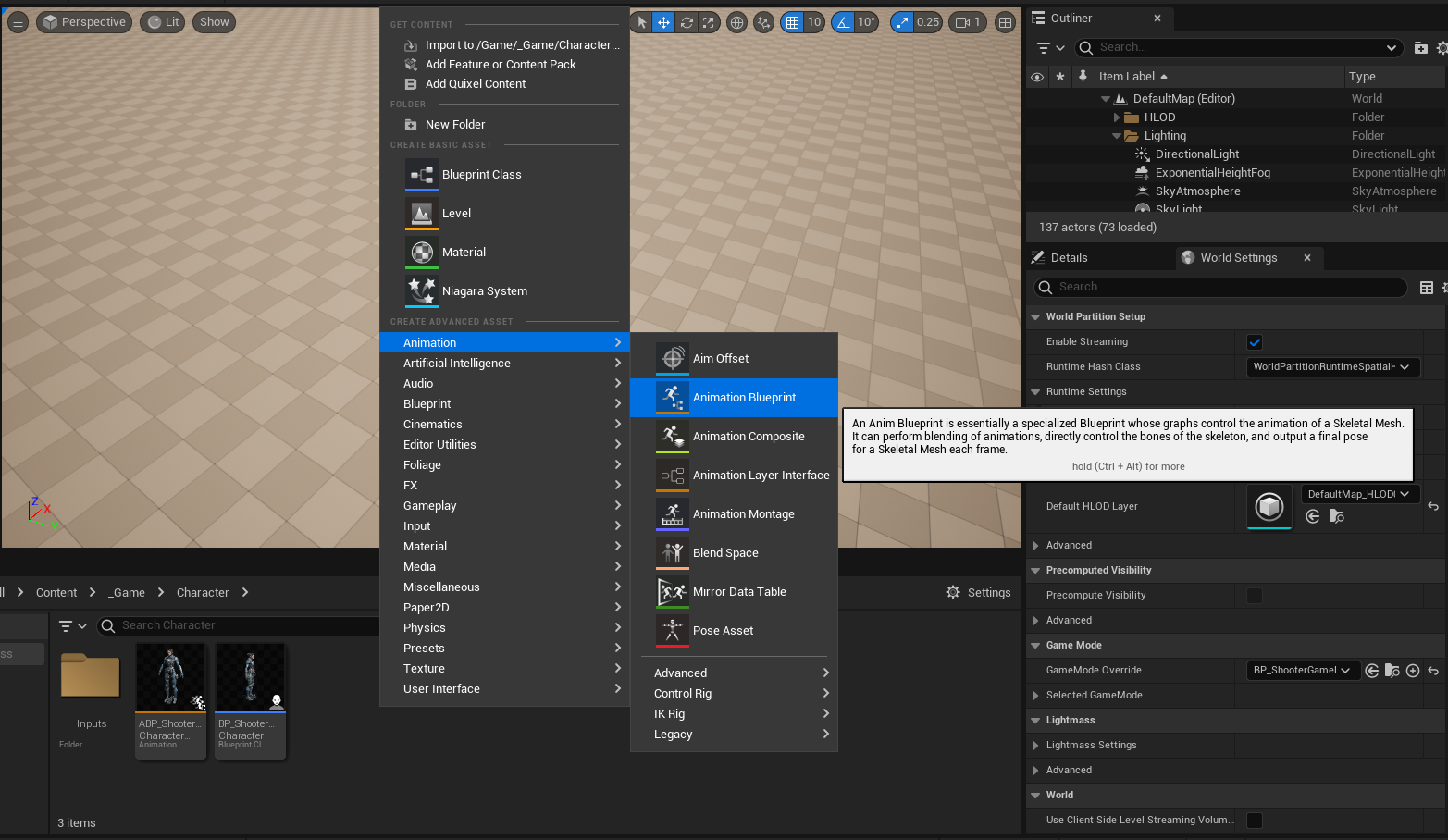
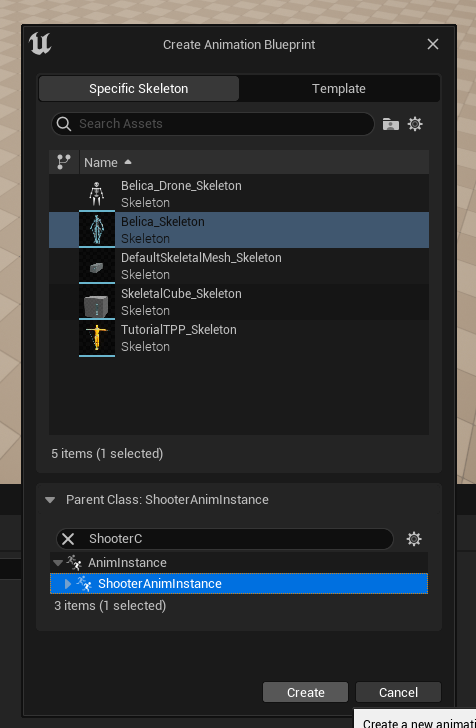
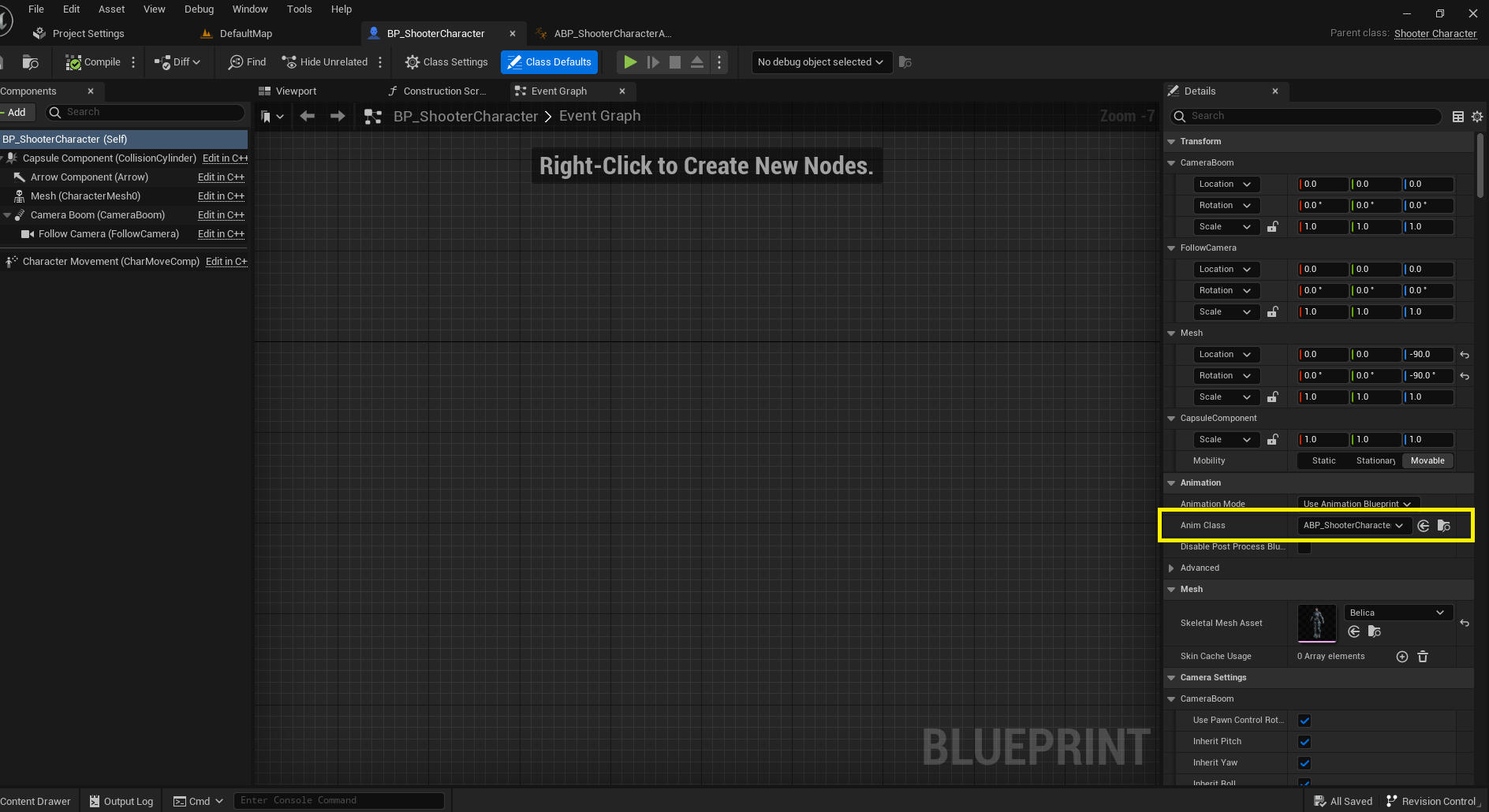
Event Graph에서 Update Animation Properties를 Event Blueprint Update Animation(애니메이션의 각 프레임을 업데이트할 때마다 자동으로 호출)과 연결

Run Animation
State Machine으로 노드를 생성합니다. (이름은 Ground Locomotion으로 수정)
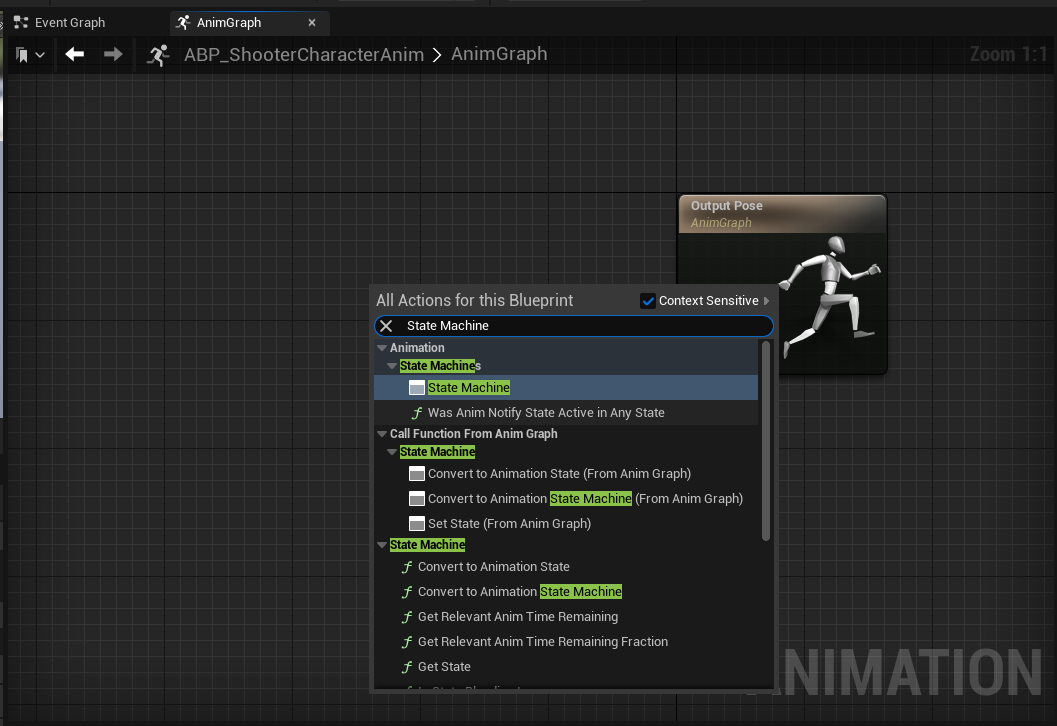
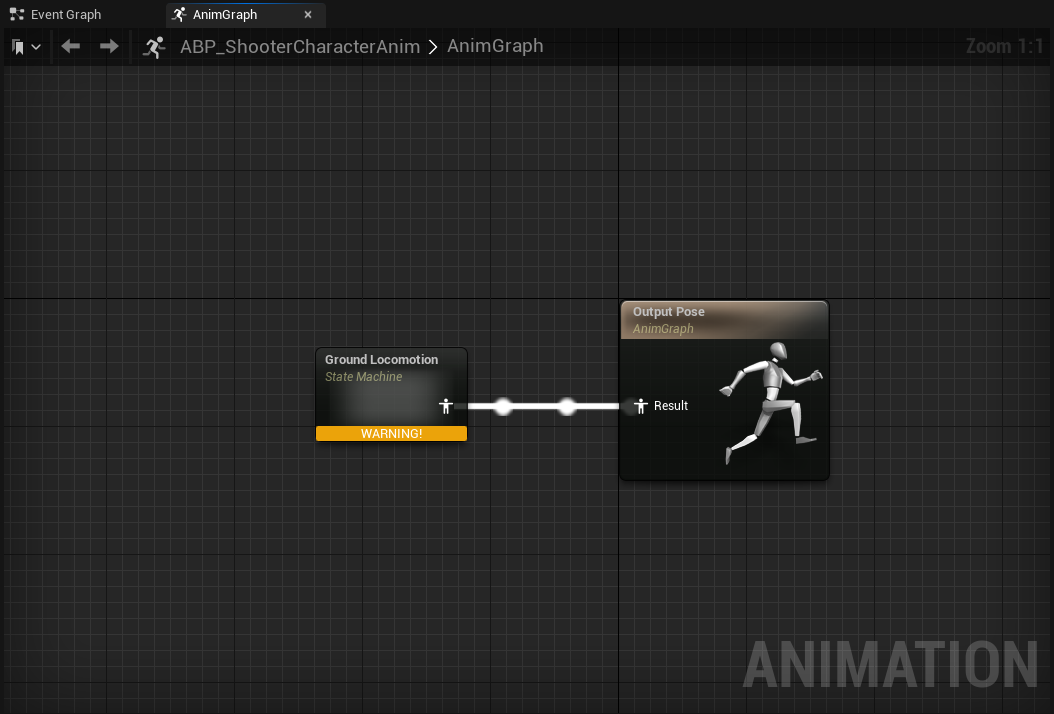
Ground Locomotion에 들어가서 Add State로 사진과 같이 그래프를 만들어 줍니다.

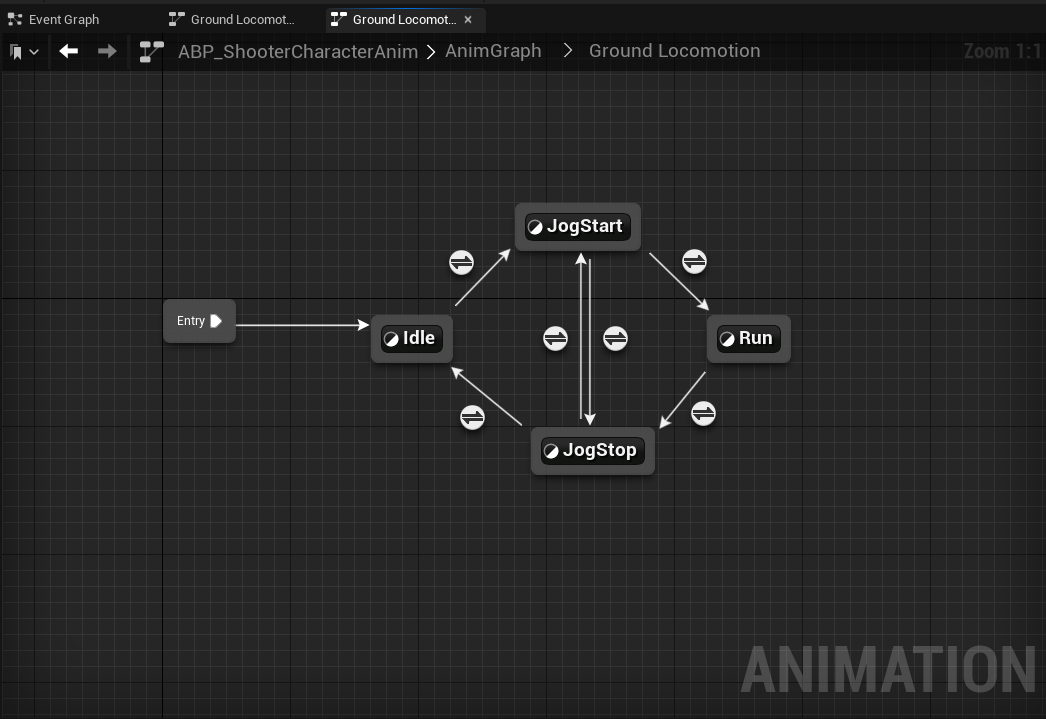
Idle State에 들어가서 좌측 하단의 Asset Browser에서 Idle_Relaced를 찾아 드래그해서 설정. 나머지는 다음 사진과 맞게 설정해 줍니다.
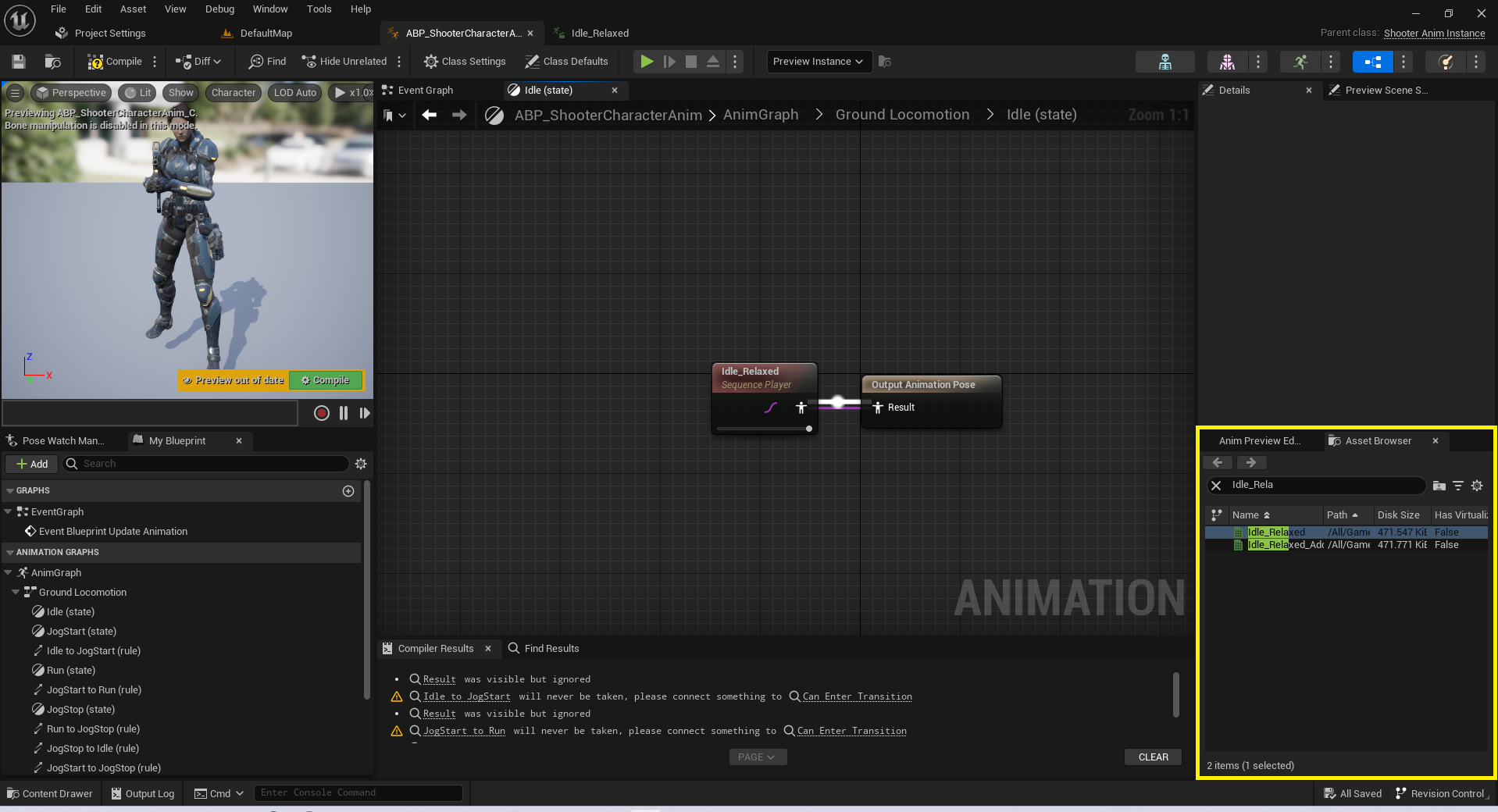
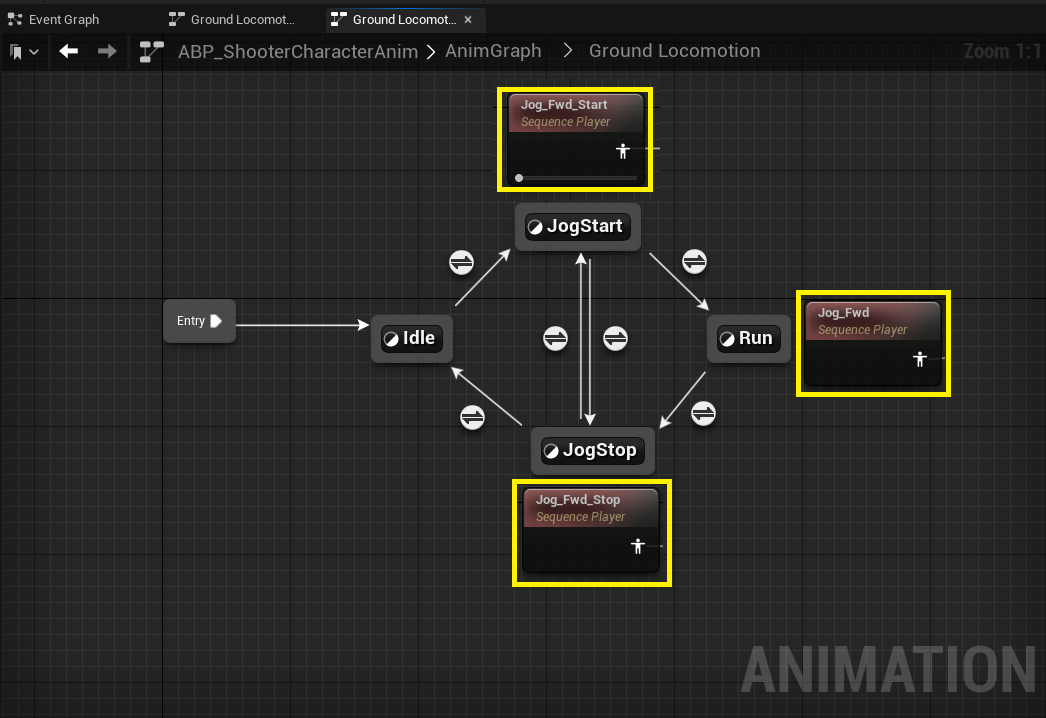
Idle과 JogStart를 연결해주는 선분위의 버튼을 눌러서 변환조건을 설정해준다.
(Speed가 0보다 크고 공중에 있지 않으며, 가속도가 있을때 달리기 시작한다.)
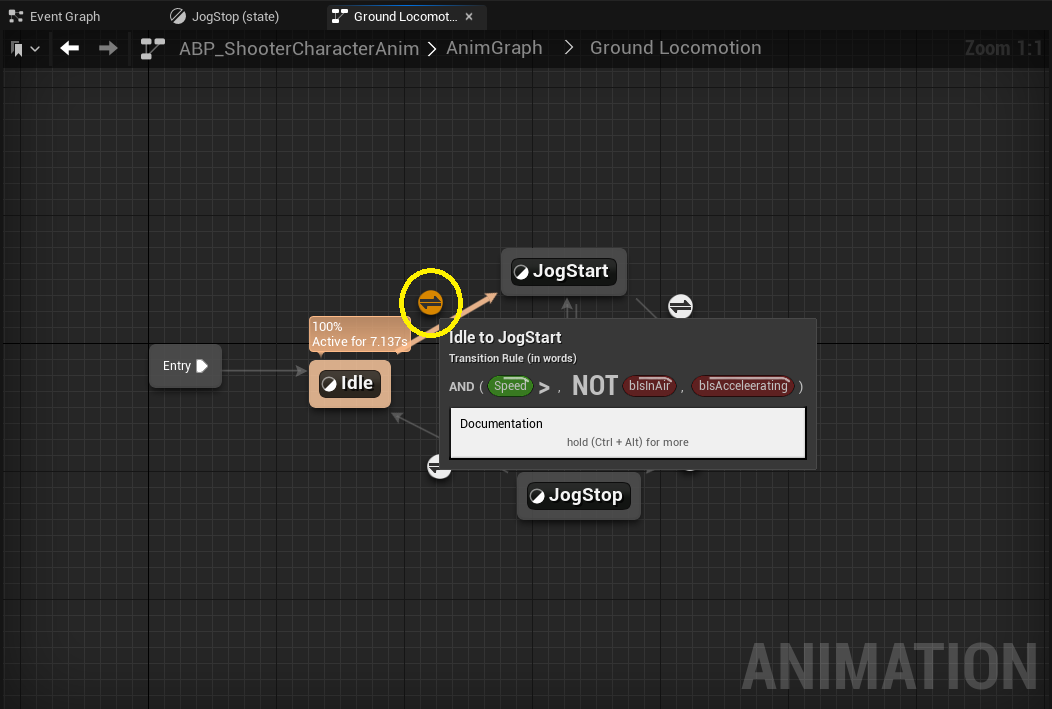
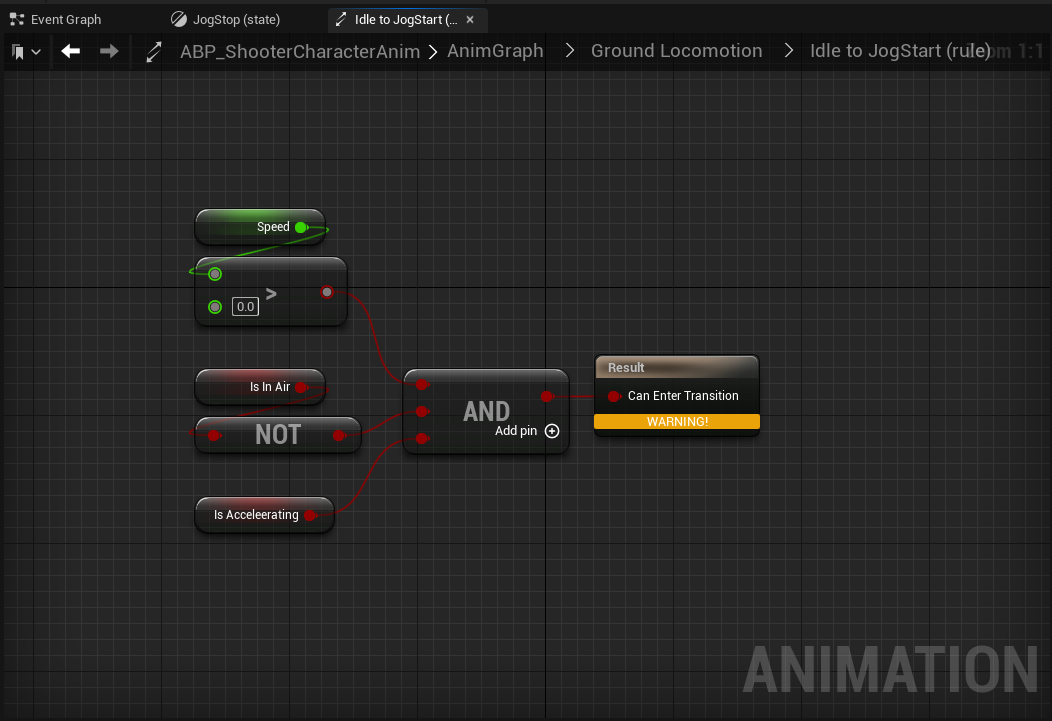
JogStart에서 Run으로 넘어가는 조건을 한번 클릭하면 Details를 수정할 수 있는데, Automatic Rule Based on Sequence Player in State를 True로 하면 애니메이션이 끝난 후 자동으로 전환된다.
(Duration은 0.8로 하는게 부드럽다는 영상의 조언에 따르겠다.)
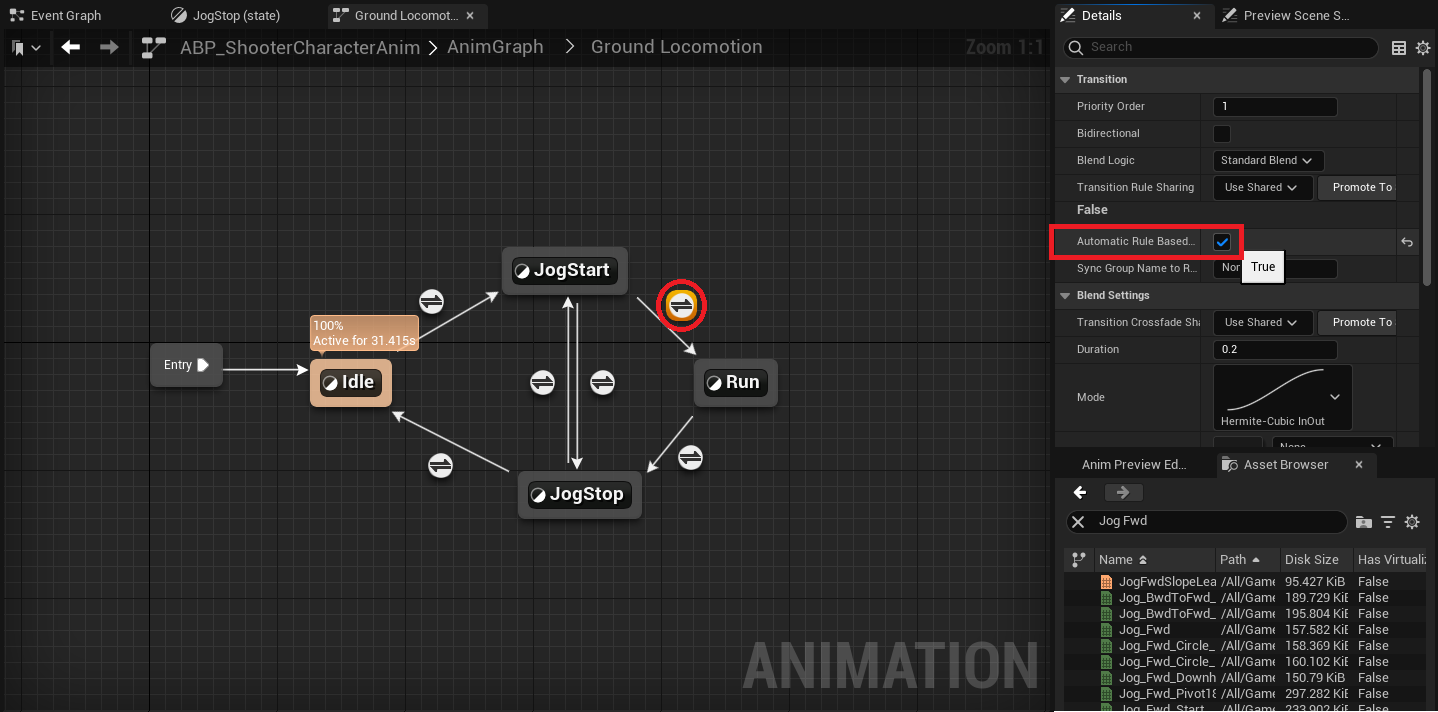
아래와 같이 설정해 줍니다.
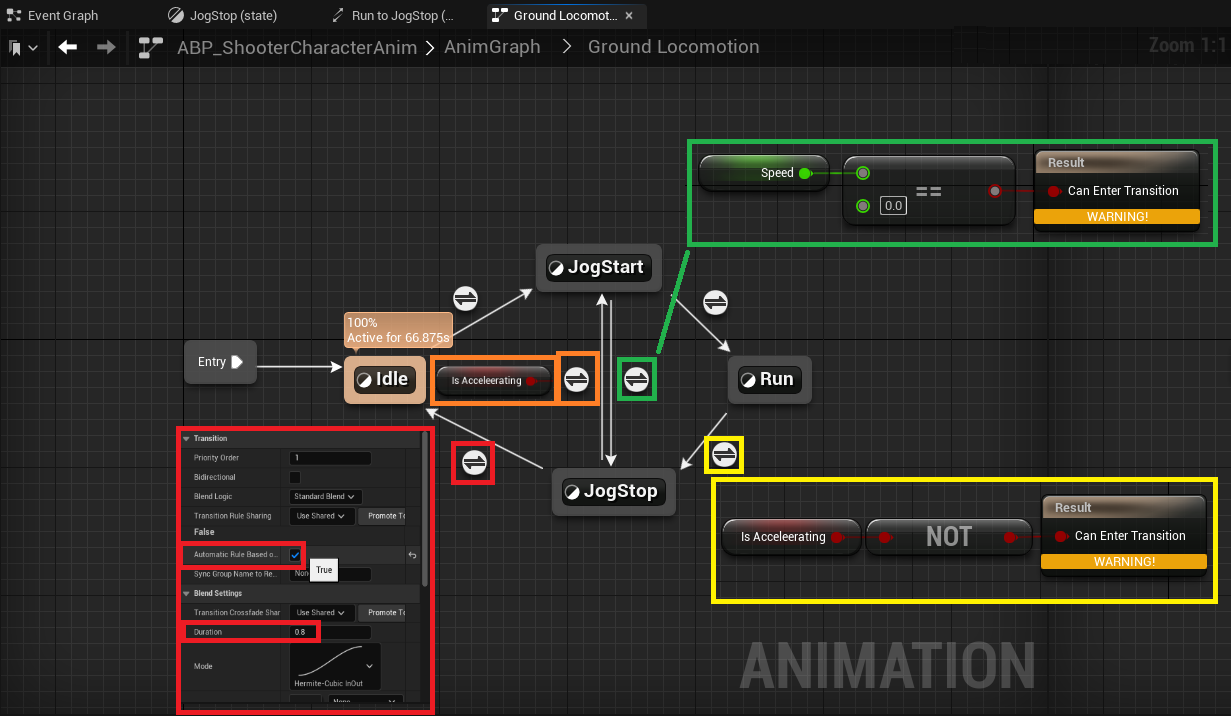
Run에 있는 Jog_Fwd에서 Detail의 Loop Animation을 True로 바꿔줘야 달리기를 계속할 수 있습니다.
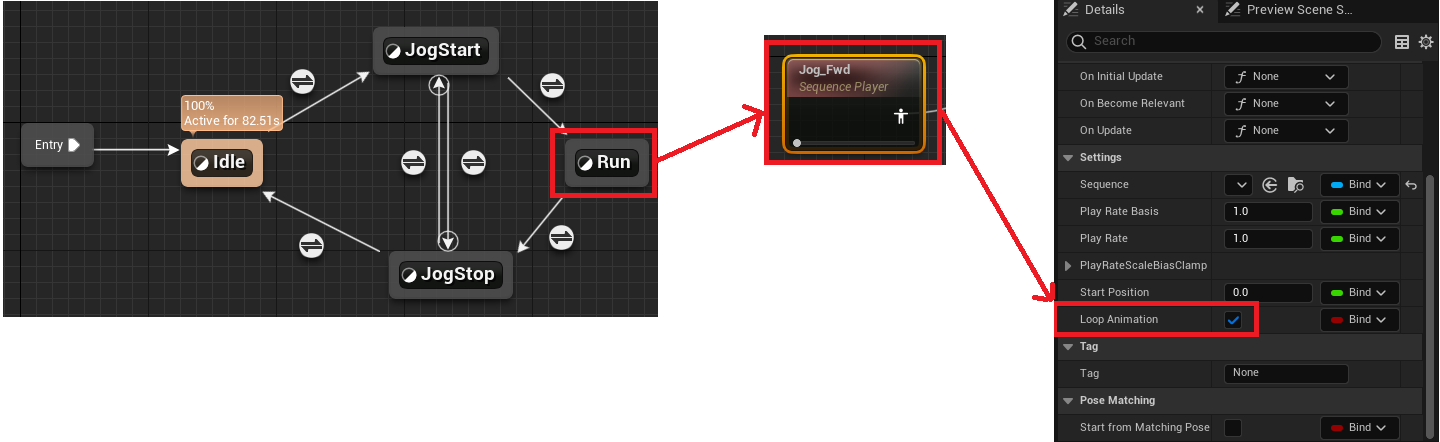
Trimming Animation
애니메이션의 수정을 할것인데 원본을 보호하기 위해서 새로운 폴더를 만들고 복사를 해줍니다.
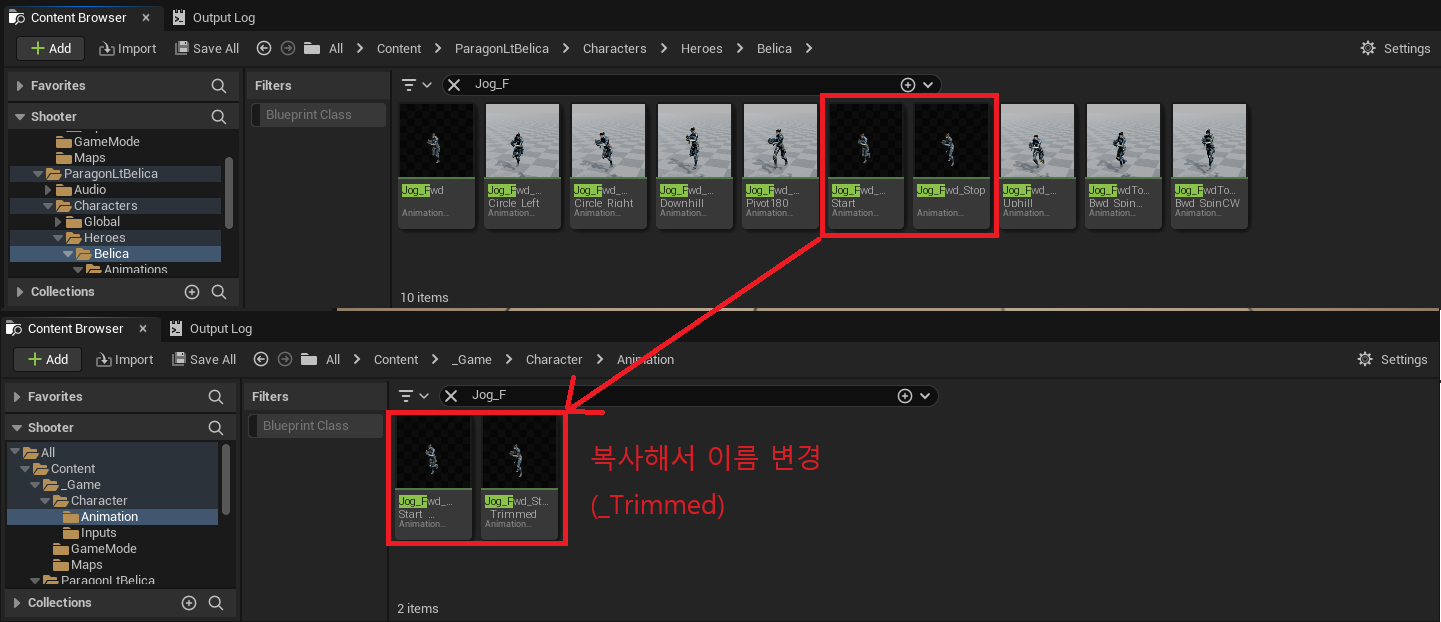
Jog_Fwd_Start_Trimmed를 수정해 줍니다.

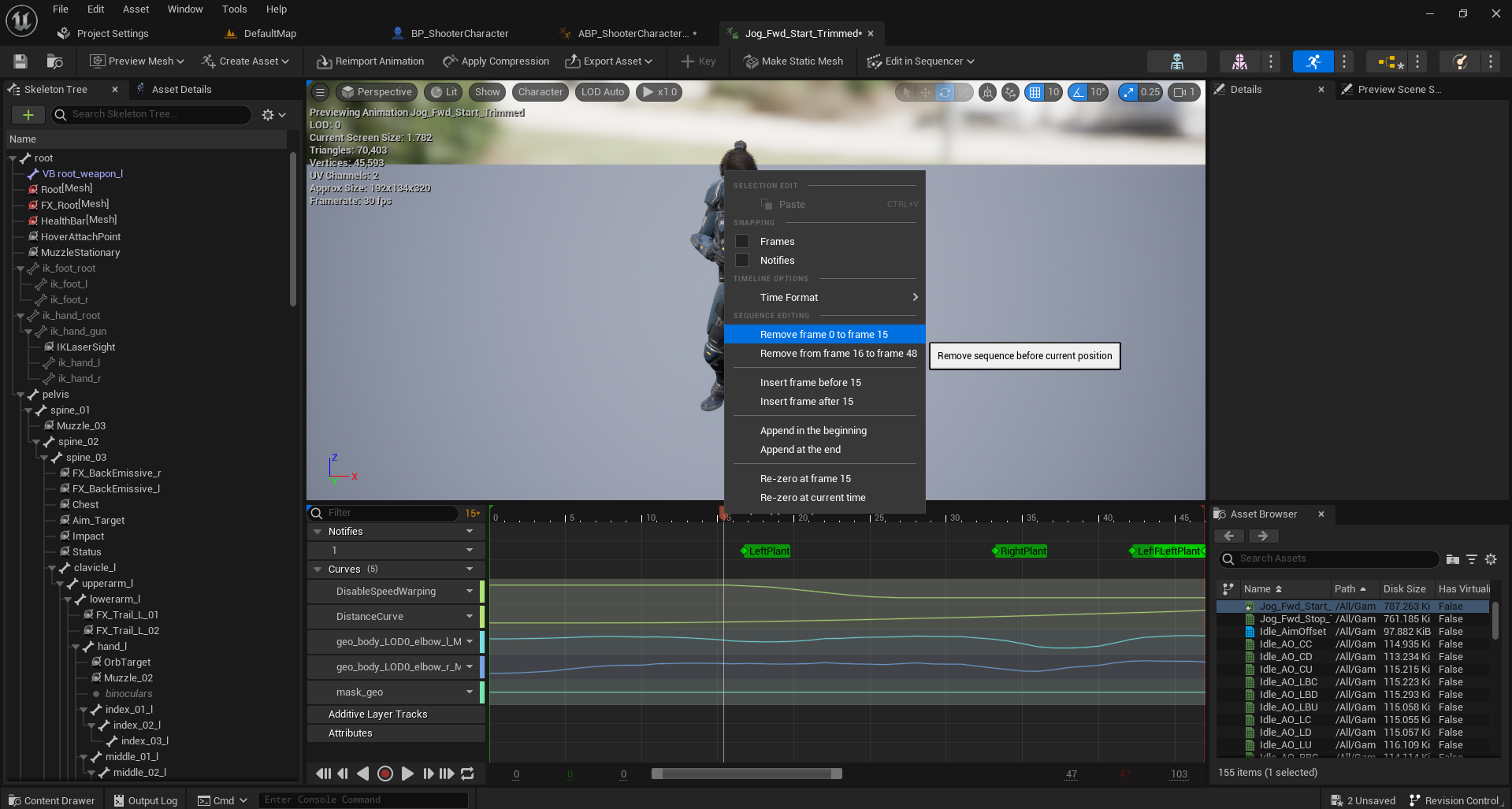
Jog_Fwd_End_Trimmed를 수정해 줍니다.
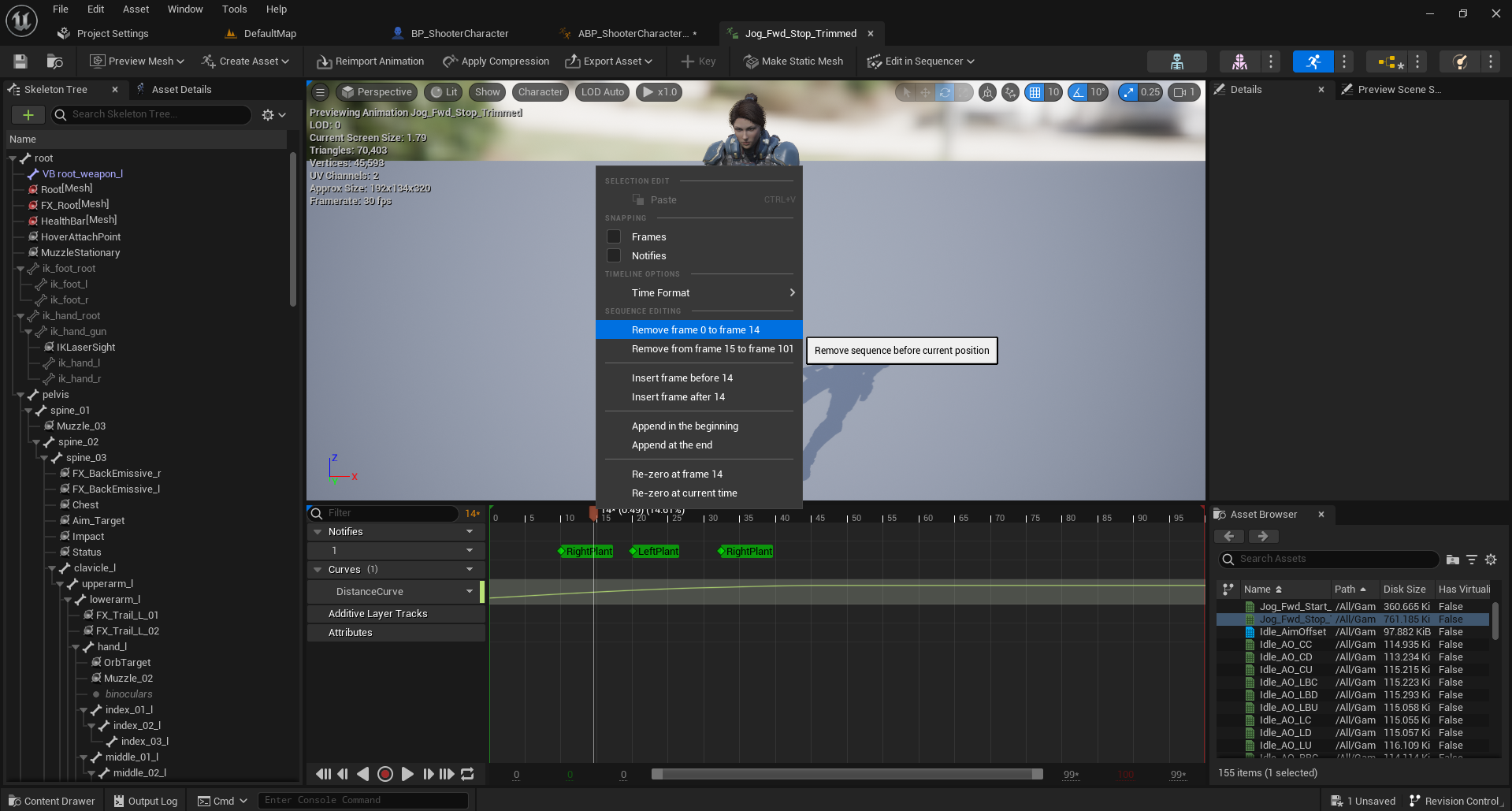
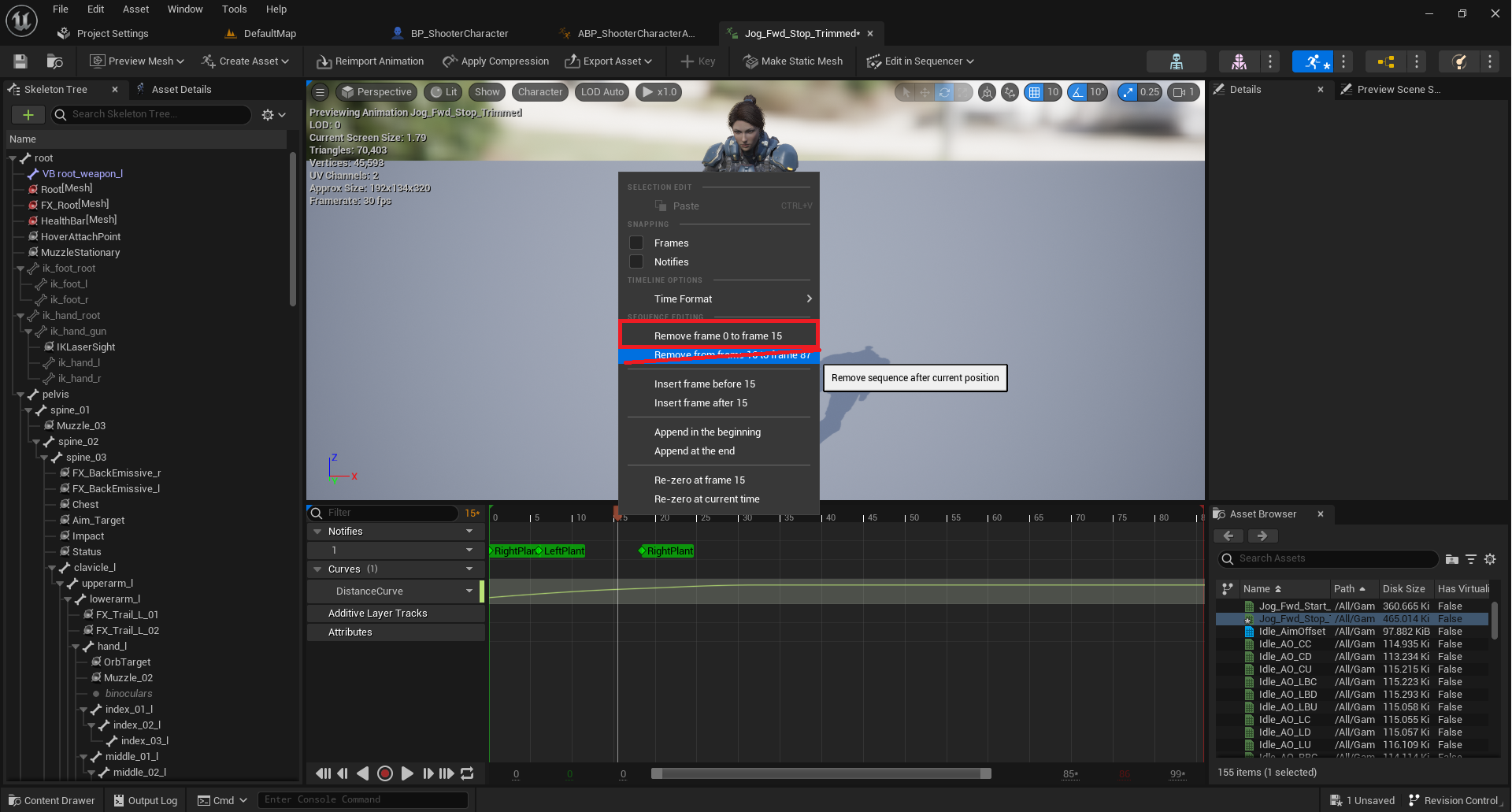
Rotate Character to Movement
ShooterCharacter.cpp 생성자에 이동 방향으로 Pawn 회전 방향을 정하고, 이동 관련 코드를 추가해줍니다.
AShooterCharacter::AShooterCharacter() : BaseTrunRate(45.f), BaseLookUpRate(45.f)
{
...
// 컨트롤러가 회전할 때 캐릭터가 회전하지 않도록 설정합니다. 컨트롤러는 오직 카메라에만 영향을 줍니다.
bUseControllerRotationPitch = false;
bUseControllerRotationYaw = false;
bUseControllerRotationRoll = false;
// 캐릭터 이동을 구성합니다
GetCharacterMovement()->bOrientRotationToMovement = true; // 캐릭터는 입력 방향으로 이동합니다
GetCharacterMovement()->RotationRate = FRotator(0.f, 540.f, 0.f); // 이 회전 속도로...
GetCharacterMovement()->JumpZVelocity = 600.f;
GetCharacterMovement()->AirControl = 0.2f;
}
- bUseControllerRotationPitch, Yaw, Roll : Pawn의 회전을 컨트롤러 회전으로 할 지 선택합니다.
- bOrientRotationToMovement : 회전 방향을 이동 방향으로 할 지 선택합니다.
- RotationRate = FRotator(0.f, 540.f, 0.f) : 캐릭터가 얼마나 빠르게 회전할 수 있는지를 정의합니다.
- 초당 540도의 속도로 Yaw 축(좌우 방향)을 중심으로 회전할 수 있음을 의미합니다.
- JumpZVelocity = 600.f : 캐릭터가 점프할 때의 수직 속도(즉, Z축 방향 속도)를 설정합니다.
- AirControl = 0.2f : 캐릭터가 공중에 있을 때 얼마나 많은 이동 제어를 할 수 있는지를 나타냅니다.
- 값이 0에 가까울수록 공중에서의 제어가 줄어들고, 1에 가까울수록 더 많은 제어를 할 수 있습니다.
- bOrientRotationToMovement와 RotationRate 설정은 캐릭터의 회전을 부드럽고 직관적으로 만들어주는 핵심 요소입니다.
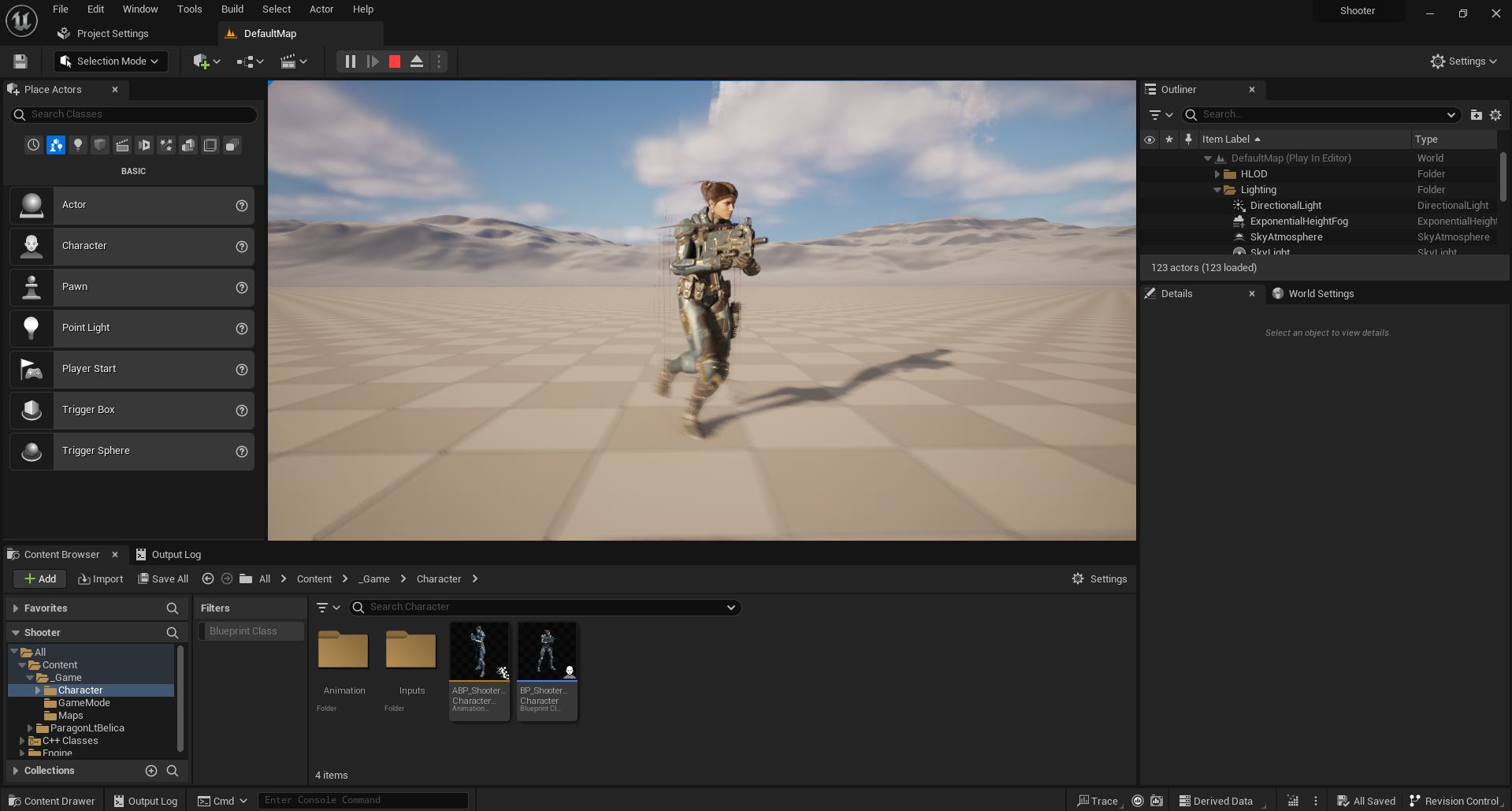
UE5를 사용하면서 회전(bOrientRotationToMovement = true)이 되지 않는 문제.
영상에서는 CPP 파일 수정후 회전이 작동하지 않을경우 BP를 확인하라 합니다. 허나 BP의 값을 수정해도 움직이지 않을경우 BP의 RotationRate 설정에 Yaw가 540으로 되어있는지 확인해 줍시다. (BP에서는 XYZ순으로 보여주기 때문에 3번째 매개변수가 540이어야 합니다.
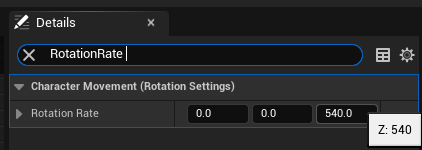
'Unreal 공부 > Unreal Engine 4 C++ The Ultimate Shooter' 카테고리의 다른 글
Animation - 5 (1) | 2023.12.31 |
---|---|
Animation - 4 (1) | 2023.12.31 |
Animation - 3 (1) | 2023.12.30 |
Animation - 2 (1) | 2023.12.28 |
[UE] C++에서 SpringArm과 Camera 구현하기 (1) | 2023.12.22 |